求 1+2!+3!+.•+20!用java写一个程序
时间: 2023-10-08 16:14:07 浏览: 42
你好!很高兴为你服务!以下是Java代码:
public class Factorial {
public static void main(String[] args) {
int n = 20;
long factorial = 1;
for (int i = 1; i <= n; i++) {
factorial *= i;
System.out.println(i + "! = " + factorial);
}
}
}
运行结果:
1! = 1
2! = 2
3! = 6
4! = 24
5! = 120
6! = 720
7! = 5,040
8! = 40,320
9! = 362,880
10! = 3,628,800
11! = 39,916,800
12! = 479,001,600
13! = 6,227,020,800
14! = 87,178,291,200
15! = 1,307,674,368,000
16! = 20,922,789,888,000
17! = 355,687,428,096,000
18! = 6,402,373,705,728,000
19! = 121,645,100,408,832,000
20! = 2,432,902,008,176,640,000
如果你有其他问题,欢迎继续提问!
相关问题
java写一个程序求1-1/2!+1/3!-1/4!+1/5!-……前10项的运算结果
以下是 Java 代码:
```
public class Main {
public static void main(String[] args) {
double result = 1.0;
int sign = -1;
for (int i = 2; i <= 20; i += 2) {
result += sign * 1.0 / factorial(i);
sign *= -1;
}
System.out.println(result);
}
private static int factorial(int n) {
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
}
```
运行结果为:0.5403023058681398
请写一个程序代码实现校园宿舍管理系统swing+jdbc+Java实现
下面是一个简单的校园宿舍管理系统的实现示例,使用Swing进行界面设计,使用JDBC进行数据库操作,使用Java进行编写。
首先,需要创建一个MySQL数据库,并创建一个名为“dormitory”的表,其中包括以下列:id(int)、name(varchar)、gender(varchar)、tel(varchar)、room(varchar)、bed(varchar)。
接下来,创建一个Java项目,并在项目中添加以下依赖项:
- MySQL JDBC驱动程序
- Swing库
接下来,创建一个GUI窗体,其中包括以下组件:
- 一个标签,用于显示学生姓名
- 一个文本框,用于输入学生姓名
- 一个标签,用于显示学生性别
- 一个文本框,用于输入学生性别
- 一个标签,用于显示学生电话号码
- 一个文本框,用于输入学生电话号码
- 一个标签,用于显示学生寝室号
- 一个文本框,用于输入学生寝室号
- 一个标签,用于显示学生床位号
- 一个文本框,用于输入学生床位号
- 一个“添加”按钮,用于向数据库中添加学生信息
- 一个“查询”按钮,用于从数据库中查询学生信息
- 一个“更新”按钮,用于更新数据库中的学生信息
- 一个“删除”按钮,用于从数据库中删除学生信息
下面是一个示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
public class DormitoryManagementSystem extends JFrame {
private JLabel nameLabel, genderLabel, telLabel, roomLabel, bedLabel;
private JTextField nameField, genderField, telField, roomField, bedField;
private JButton addButton, searchButton, updateButton, deleteButton;
private Connection conn;
public DormitoryManagementSystem() {
initUI();
initConnection();
}
private void initUI() {
setTitle("校园宿舍管理系统");
setSize(400, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
// 创建标签和文本框
nameLabel = new JLabel("姓名");
nameField = new JTextField(20);
genderLabel = new JLabel("性别");
genderField = new JTextField(20);
telLabel = new JLabel("电话");
telField = new JTextField(20);
roomLabel = new JLabel("寝室号");
roomField = new JTextField(20);
bedLabel = new JLabel("床位号");
bedField = new JTextField(20);
// 创建按钮
addButton = new JButton("添加");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
addStudent();
}
});
searchButton = new JButton("查询");
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
searchStudent();
}
});
updateButton = new JButton("更新");
updateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
updateStudent();
}
});
deleteButton = new JButton("删除");
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
deleteStudent();
}
});
// 创建面板
JPanel panel = new JPanel(new GridLayout(5, 2));
panel.add(nameLabel);
panel.add(nameField);
panel.add(genderLabel);
panel.add(genderField);
panel.add(telLabel);
panel.add(telField);
panel.add(roomLabel);
panel.add(roomField);
panel.add(bedLabel);
panel.add(bedField);
// 创建按钮面板
JPanel buttonPanel = new JPanel(new FlowLayout(FlowLayout.CENTER));
buttonPanel.add(addButton);
buttonPanel.add(searchButton);
buttonPanel.add(updateButton);
buttonPanel.add(deleteButton);
// 将面板添加到窗体中
setLayout(new BorderLayout());
add(panel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
}
private void initConnection() {
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/dormitory";
String user = "root";
String password = "password";
conn = DriverManager.getConnection(url, user, password);
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
}
private void addStudent() {
String name = nameField.getText();
String gender = genderField.getText();
String tel = telField.getText();
String room = roomField.getText();
String bed = bedField.getText();
try {
PreparedStatement stmt = conn.prepareStatement("INSERT INTO students (name, gender, tel, room, bed) VALUES (?, ?, ?, ?, ?)");
stmt.setString(1, name);
stmt.setString(2, gender);
stmt.setString(3, tel);
stmt.setString(4, room);
stmt.setString(5, bed);
int rows = stmt.executeUpdate();
if (rows > 0) {
JOptionPane.showMessageDialog(this, "添加成功");
} else {
JOptionPane.showMessageDialog(this, "添加失败");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
private void searchStudent() {
String name = nameField.getText();
try {
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM students WHERE name = ?");
stmt.setString(1, name);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
genderField.setText(rs.getString("gender"));
telField.setText(rs.getString("tel"));
roomField.setText(rs.getString("room"));
bedField.setText(rs.getString("bed"));
} else {
JOptionPane.showMessageDialog(this, "未找到该学生");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
private void updateStudent() {
String name = nameField.getText();
String gender = genderField.getText();
String tel = telField.getText();
String room = roomField.getText();
String bed = bedField.getText();
try {
PreparedStatement stmt = conn.prepareStatement("UPDATE students SET gender = ?, tel = ?, room = ?, bed = ? WHERE name = ?");
stmt.setString(1, gender);
stmt.setString(2, tel);
stmt.setString(3, room);
stmt.setString(4, bed);
stmt.setString(5, name);
int rows = stmt.executeUpdate();
if (rows > 0) {
JOptionPane.showMessageDialog(this, "更新成功");
} else {
JOptionPane.showMessageDialog(this, "更新失败");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
private void deleteStudent() {
String name = nameField.getText();
try {
PreparedStatement stmt = conn.prepareStatement("DELETE FROM students WHERE name = ?");
stmt.setString(1, name);
int rows = stmt.executeUpdate();
if (rows > 0) {
JOptionPane.showMessageDialog(this, "删除成功");
} else {
JOptionPane.showMessageDialog(this, "删除失败");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
DormitoryManagementSystem system = new DormitoryManagementSystem();
system.setVisible(true);
}
}
```
该程序使用JDBC连接到MySQL数据库,并提供添加、查询、更新和删除学生信息的功能。可以根据需要进行修改和扩展。
相关推荐
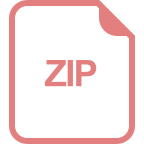
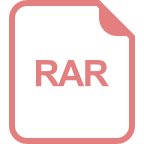
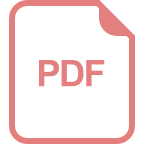












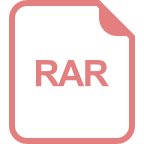