qt 如何设计当鼠标处于Y轴范围时可以自由拖动Y轴
时间: 2023-10-06 20:06:01 浏览: 32
在 Qt 中,你可以使用 QGraphicsView 和 QGraphicsScene 类来实现当鼠标处于 Y 轴范围时可以自由拖动 Y 轴的功能。你可以通过以下步骤来实现:
1. 创建 QGraphicsView 和 QGraphicsScene 对象,将 QGraphicsScene 设置为 QGraphicsView 的场景。
2. 创建一个 QGraphicsLineItem 对象,将其添加到 QGraphicsScene 中。这个 QGraphicsLineItem 对象表示 Y 轴。
3. 重写 QGraphicsView 的 mousePressEvent 和 mouseMoveEvent 事件处理函数,以便在鼠标按下并移动时拖动 Y 轴。
在 mousePressEvent 事件处理函数中,你需要检查鼠标是否在 Y 轴范围内。如果是,设置一个标志,以便在 mouseMoveEvent 中可以拖动 Y 轴。
在 mouseMoveEvent 事件处理函数中,你需要检查标志并计算鼠标移动的距离。然后,将 Y 轴的位置调整相应的距离。
最后,在 mouseReleaseEvent 事件处理函数中,你需要清除标志。
下面是一个简单的示例代码,可以帮助你开始实现这个功能:
```cpp
class MyView : public QGraphicsView
{
public:
MyView(QWidget* parent = nullptr) : QGraphicsView(parent)
{
// 创建 QGraphicsScene 对象并设置为场景
scene = new QGraphicsScene(this);
setScene(scene);
// 创建 Y 轴 QGraphicsLineItem 对象
line = new QGraphicsLineItem(QLineF(0, 0, 0, 200));
line->setPen(QPen(Qt::black, 2));
scene->addItem(line);
}
protected:
void mousePressEvent(QMouseEvent* event) override
{
// 检查鼠标是否在 Y 轴范围内
QPointF pos = mapToScene(event->pos());
if (pos.x() >= -5 && pos.x() <= 5)
{
// 设置标志,允许拖动 Y 轴
dragging = true;
lastPos = pos;
}
else
{
QGraphicsView::mousePressEvent(event);
}
}
void mouseMoveEvent(QMouseEvent* event) override
{
if (dragging)
{
// 计算鼠标移动的距离
QPointF delta = mapToScene(event->pos()) - lastPos;
// 调整 Y 轴的位置
line->setLine(line->line().translated(delta.x(), delta.y()));
// 更新 lastPos
lastPos = mapToScene(event->pos());
}
else
{
QGraphicsView::mouseMoveEvent(event);
}
}
void mouseReleaseEvent(QMouseEvent* event) override
{
if (dragging)
{
// 清除标志
dragging = false;
}
else
{
QGraphicsView::mouseReleaseEvent(event);
}
}
private:
QGraphicsScene* scene;
QGraphicsLineItem* line;
bool dragging = false;
QPointF lastPos;
};
```
这只是一个简单的示例代码,可以帮助你开始实现这个功能。你可以根据自己的需求进行修改和扩展。
相关推荐
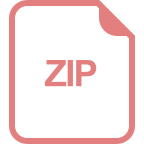
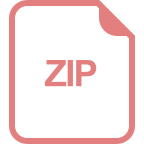














