#include <iostream> using namespace std; #define MVNum 100 typedef int Status; typedef char VerTexType; typedef int ArcType; typedef int OtherInfo; typedef struct ArcNode{ int adjvex; struct ArcNode *nextarc; OtherInfo info; }ArcNode; typedef struct VNode{ VerTexType data; ArcNode *firstarc; }VNode,AdjList[MVNum]; typedef struct { AdjList vertices; //vertex的复数为vertices int vexnum,arcnum; }ALGraph; int LocateVex(ALGraph G,char u) { /* 初始条件: 图G存在,u和G中顶点有相同特征*/ /* 操作结果: 若G中存在顶点u,则返回该顶点在图中位置;否则返回-1 */ for(int i=0;i<G.vexnum;++i){ if(u==G.vertices[i].data){ return i; } } return -1; } Status CreateUDG(ALGraph &G){ int i,j,k; char v1,v2; cin>>G.vexnum>>G.arcnum; for(i=0;i<G.vexnum;++i){ cin>>G.vertices[i].data; G.vertices[i].firstarc=NULL; } for (k=0;k<G.arcnum;++k){ cin>>v1>>v2; i=LocateVex(G,v1); j=LocateVex(G,v2); ArcNode *p1,*p2; p1=new ArcNode;p1->adjvex=j; p1->nextarc=G.vertices[i].firstarc; G.vertices[i].firstarc=p1; p2=new ArcNode;p2->adjvex=i; p2->nextarc=G.vertices[j].firstarc; G.vertices[j].firstarc=p2; } return 0; } int main{ CreateUDG(ALGraph H) return 0; }
时间: 2024-04-09 14:30:49 浏览: 50
这是一个创建无向图的程序。程序中定义了一些结构体和类型别名,包括顶点结构体VNode和边结构体ArcNode。CreateUDG函数用于创建无向图,根据输入的顶点数和边数,以及每条边的起始顶点和终止顶点,构建图的邻接表表示。LocateVex函数用于查找指定顶点在图中的位置。
在主函数中,调用CreateUDG函数创建一个无向图。请问有什么问题我可以帮助你解答的吗?
相关问题
#include <iostream> #include <fstream> using namespace std; #define MVNum 100//最大顶点数 typedef char VerTexType;//顶点的数据类型为字符型 typedef int ArcType;//边的权值类型为整型 //图的邻接矩阵 typedef struct{ … return 0; }
这段代码不完整,缺少结构体的定义和函数的实现。可以简单解释一下代码的意思:
1. 定义了一个最大顶点数为100的常量MVNum,表示图中顶点的最大数量。
2. 定义了顶点的数据类型为字符型,边的权值类型为整型。
3. 接下来应该是定义图的邻接矩阵的结构体,但是这部分代码没有给出。
4. 最后定义了一个main函数,但是函数体没有写完。
顺序表插入操作 #include<iostream> using namespace std; #
顺序表插入操作是指在顺序表的第i个位置插入元素e,并将原来位于第i个位置及其之后的元素都后移一个位置。具体实现可以使用一个循环将第i个位置及其之后的元素后移,然后将元素e插入到第i个位置。以下是一个C++实现的示例代码:
```
#include<iostream>
using namespace std;
#define OK 1
#define ERROR 0
#define MAXSIZE 100
typedef int datatype;
typedef struct {
datatype *elem;
int length;
} SqList;
int ListInsert_Sq(SqList &L, int i, datatype e) {
if ((i < 1) || (i > L.length + 1)) {
return ERROR;
}
if (L.length >= MAXSIZE) {
return ERROR;
}
for (int j = L.length; j >= i; j--) {
L.elem[j] = L.elem[j - 1];
}
L.elem[i - 1] = e;
L.length++;
return OK;
}
```
--相关问题--:
阅读全文
相关推荐
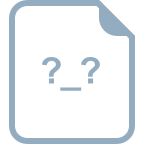






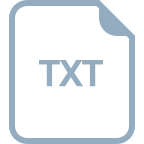

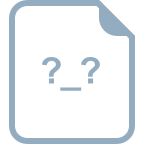
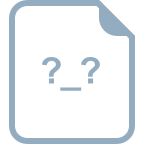
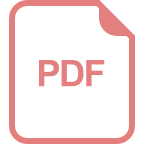


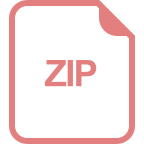
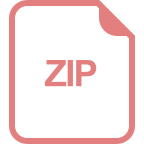