c语言来建立一个树从树上找任意两个结点最近的公共祖并输出先完整代码
时间: 2024-05-13 16:15:24 浏览: 17
以下是使用C语言建立树并找到任意两个结点最近公共祖先的完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义树的节点结构体
struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
};
// 创建树的节点函数
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// 在树中查找指定节点,并返回其指针
struct TreeNode* findNode(struct TreeNode* root, int val) {
if (root == NULL) {
return NULL;
}
if (root->val == val) {
return root;
}
struct TreeNode* left = findNode(root->left, val);
struct TreeNode* right = findNode(root->right, val);
if (left != NULL) {
return left;
}
if (right != NULL) {
return right;
}
return NULL;
}
// 查找任意两个节点的最近公共祖先
struct TreeNode* lowestCommonAncestor(struct TreeNode* root, struct TreeNode* p, struct TreeNode* q) {
if (root == NULL || root == p || root == q) {
return root;
}
struct TreeNode* left = lowestCommonAncestor(root->left, p, q);
struct TreeNode* right = lowestCommonAncestor(root->right, p, q);
if (left != NULL && right != NULL) {
return root;
}
if (left != NULL) {
return left;
}
if (right != NULL) {
return right;
}
return NULL;
}
// 主函数
int main() {
// 创建树
struct TreeNode* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
// 查找任意两个节点的最近公共祖先并输出
struct TreeNode* node1 = findNode(root, 4);
struct TreeNode* node2 = findNode(root, 5);
struct TreeNode* lca = lowestCommonAncestor(root, node1, node2);
printf("The lowest common ancestor of %d and %d is %d.\n", node1->val, node2->val, lca->val);
// 释放内存
free(root->left->right);
free(root->left->left);
free(root->right->right);
free(root->right->left);
free(root->left);
free(root->right);
free(root);
return 0;
}
```
在这个例子中,我们创建了一个树,然后在树中找到了值为4和5的两个节点,最后找到了它们的最近公共祖先并输出。你可以根据需要修改这个代码,以适应你的具体需求。
相关推荐
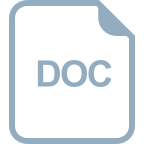
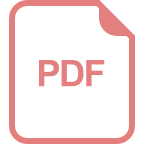
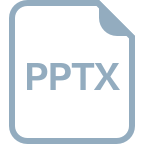














