用python opencv写一段代码用来批量读取一个文件夹中的所有图片
时间: 2023-03-08 09:08:26 浏览: 119
答: 使用python opencv读取文件夹中图片的代码如下:import os
import cv2# 设置文件夹路径
folder_path = '文件夹路径'# 循环遍历文件夹中的图片
for image_path in os.listdir(folder_path):
img = cv2.imread(os.path.join(folder_path, image_path))
# 对图片进行处理(这部分可以根据实际需求自行编写)
# ...
# ...
# ...
# 保存图片
cv2.imwrite('输出路径/{}'.format(image_path), img)
相关问题
用python写一段程序:批量提取文件夹及子文件夹中图片上的文字和数据,并检查其中经纬度的正确性
下面是一段使用 Python 的代码,实现了批量提取文件夹及子文件夹中图片上的文字和数据,并检查其中经纬度的正确性:
```
import os
import cv2
import pytesseract
def extract_text_from_image(image_path):
# 使用 OpenCV 读取图片
image = cv2.imread(image_path)
# 使用 pytesseract 识别图片中的文字
text = pytesseract.image_to_string(image)
return text
def check_lat_long(text):
# 在这里写代码来检查经纬度的正确性
pass
def extract_text_from_folder(folder_path):
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith(".jpg") or file.endswith(".png"):
image_path = os.path.join(root, file)
text = extract_text_from_image(image_path)
check_lat_long(text)
# 使用示例
folder_path = "path/to/your/folder"
extract_text_from_folder(folder_path)
```
请注意,这段代码需要依赖 OpenCV 和 pytesseract 这两个库。因此,请确保在使用前安装了这两个库。
python opencv 批量处理图片代码
很高兴回答你的问题,以下是一段 Python OpenCV 批量处理图片的代码示例:
```
import cv2
import glob
# 获取文件夹内所有图片文件的路径
img_paths = glob.glob('images/*.jpg')
for img_path in img_paths:
# 读取图片
img = cv2.imread(img_path)
# 处理图片,例如调整亮度、裁剪等操作
# ...
# 保存处理后的图片
cv2.imwrite('processed_images/' + img_path.split('/')[-1], img)
```
希望对你有所帮助!
相关推荐
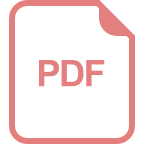
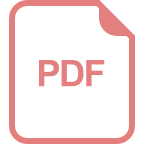
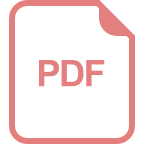
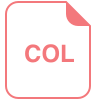
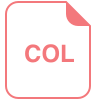
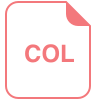
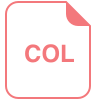
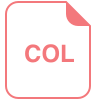








