python求学生考试的总分total和平均分average
时间: 2024-05-08 22:14:58 浏览: 10
假设有一个学生考了三门课,分别得到了80,90和70分,可以使用以下python代码计算总分和平均分:
```python
scores = [80, 90, 70] # 学生考试成绩列表
total = sum(scores) # 计算总分
average = total / len(scores) # 计算平均分
print("总分为:", total)
print("平均分为:", average)
```
输出结果为:
```
总分为: 240
平均分为: 80.0
```
注意,这个代码假设成绩已经存储在一个列表中(即`scores`),如果需要从文件或者其他数据源中读取成绩,需要先将数据读入到一个列表或者数组中。
相关问题
用python求六门考试总分和平均分
好的,我可以为您提供Python代码来求六门考试的总分和平均分。假设六门考试的分数分别为:math_score, english_score, history_score, science_score, art_score, music_score。可以使用以下代码来计算总分和平均分:
```python
# 六门考试分数
math_score = 80
english_score = 90
history_score = 85
science_score = 92
art_score = 78
music_score = 88
# 总分
total_score = math_score + english_score + history_score + science_score + art_score + music_score
print("六门考试总分为:", total_score)
# 平均分
average_score = total_score / 6
print("六门考试平均分为:", average_score)
```
请根据您的具体情况修改代码中的分数值。
用python统计一个学生的总分和平均分
### 回答1:
你好,关于你的问题,我可以回答。使用 Python 统计一个学生的总分和平均分,可以先定义一个列表,将学生的每个科目成绩存入列表中,然后使用 sum() 函数求出总分,再除以科目数求出平均分。具体代码如下:
```python
scores = [85, 90, 92, 88, 95] # 学生的各科成绩
total_score = sum(scores) # 总分
average_score = total_score / len(scores) # 平均分
print("总分为:", total_score)
print("平均分为:", average_score)
```
希望能帮到你。
### 回答2:
要用Python统计一个学生的总分和平均分,首先需要获得学生的成绩列表。可以用一个包含成绩的列表或者字典来表示学生的成绩。
假设我们有一个包含学生成绩的列表,可以按照以下步骤来计算总分和平均分:
1. 首先,定义一个表示学生成绩的列表,列表中每个元素代表一个科目的成绩。
```python
scores = [90, 85, 92, 88, 95]
```
2. 使用Python内置函数`sum()`计算成绩的总和。
```python
total = sum(scores)
```
3. 使用内置函数`len()`计算成绩的科目总数,即列表中元素的个数。
```python
num_subjects = len(scores)
```
4. 计算平均分,将总和除以科目数量。
```python
average = total / num_subjects
```
5. 最后,我们可以打印出总分和平均分。
```python
print("总分:", total)
print("平均分:", average)
```
这样,我们就可以用Python统计一个学生的总分和平均分了。记得提前定义好成绩的列表,并对每个步骤进行适当的调整。
### 回答3:
使用Python可以很方便地统计一个学生的总分和平均分。下面是一个简单的示例代码:
```
# 学生姓名
student_name = input("请输入学生姓名:")
# 学生各科成绩
scores = []
subject_names = ["语文", "数学", "英语", "物理", "化学"] # 假设有五门科目
# 输入学生各科成绩
for subject_name in subject_names:
score = float(input("请输入{}成绩:".format(subject_name)))
scores.append(score)
# 计算总分
total_score = sum(scores)
# 计算平均分
average_score = total_score / len(scores)
print("=========================")
print("学生姓名:{}".format(student_name))
for i in range(len(subject_names)):
print("{}成绩:{}".format(subject_names[i], scores[i]))
print("-------------------------")
print("总分:{}".format(total_score))
print("平均分:{:.2f}".format(average_score))
```
运行以上代码后,程序会要求用户输入学生的姓名和各科成绩,然后计算总分和平均分,并将结果输出。请注意,以上代码仅为简单示例,未考虑输入的合法性及异常处理,实际使用时还需根据实际情况进行相应的改进。
相关推荐
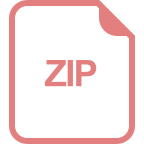













