用java web代码设计一个基于MVC设计模式的点餐系统,该系统可以供用户点餐,包含的功能有: (1)显示菜品的相关信息(价格、原材料产地和生产日期等) (2)菜品加入购物车 (3)菜品从购物车删除 (4)清空购物车 (5)计算购物车中菜品的总金额
时间: 2024-02-02 14:05:24 浏览: 21
好的,我可以为您提供一个简单的基于MVC设计模式的点餐系统的Java Web代码,以下是一个简单的实现:
首先,我们需要创建一个Model类来处理数据:
```
public class MenuItem {
private String name;
private double price;
private String origin;
private String productionDate;
public MenuItem(String name, double price, String origin, String productionDate) {
this.name = name;
this.price = price;
this.origin = origin;
this.productionDate = productionDate;
}
// getters and setters
}
```
接下来,我们需要创建一个Controller类来处理用户请求:
```
public class MenuController {
private List<MenuItem> menuItems;
private List<MenuItem> cartItems;
public MenuController() {
menuItems = new ArrayList<>();
cartItems = new ArrayList<>();
// Add some sample items to the menu
menuItems.add(new MenuItem("Hamburger", 5.99, "USA", "2022-01-01"));
menuItems.add(new MenuItem("French Fries", 2.99, "USA", "2022-01-01"));
menuItems.add(new MenuItem("Chicken Nuggets", 4.99, "USA", "2022-01-01"));
}
public List<MenuItem> getMenuItems() {
return menuItems;
}
public List<MenuItem> getCartItems() {
return cartItems;
}
public void addToCart(int index) {
cartItems.add(menuItems.get(index));
}
public void removeFromCart(int index) {
cartItems.remove(index);
}
public void clearCart() {
cartItems.clear();
}
public double calculateTotal() {
double total = 0.0;
for (MenuItem item : cartItems) {
total += item.getPrice();
}
return total;
}
}
```
最后,我们需要创建一个View类来显示数据和处理用户输入:
```
public class MenuView extends HttpServlet {
private MenuController controller;
@Override
public void init() throws ServletException {
controller = new MenuController();
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Display the menu
request.setAttribute("menuItems", controller.getMenuItems());
request.getRequestDispatcher("/menu.jsp").forward(request, response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
// Handle user input
switch (action) {
case "add":
int index = Integer.parseInt(request.getParameter("index"));
controller.addToCart(index);
break;
case "remove":
int cartIndex = Integer.parseInt(request.getParameter("cartIndex"));
controller.removeFromCart(cartIndex);
break;
case "clear":
controller.clearCart();
break;
}
// Display the cart
request.setAttribute("cartItems", controller.getCartItems());
request.setAttribute("total", controller.calculateTotal());
request.getRequestDispatcher("/cart.jsp").forward(request, response);
}
}
```
最后,我们需要创建两个JSP页面,用于显示菜单和购物车:
menu.jsp:
```
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Menu</title>
</head>
<body>
<h1>Menu</h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
<th>Origin</th>
<th>Production Date</th>
<th></th>
</tr>
</thead>
<tbody>
<c:forEach items="${menuItems}" var="item" varStatus="status">
<tr>
<td>${item.name}</td>
<td>${item.price}</td>
<td>${item.origin}</td>
<td>${item.productionDate}</td>
<td>
<form method="post" action="/menu">
<input type="hidden" name="action" value="add" />
<input type="hidden" name="index" value="${status.index}" />
<input type="submit" value="Add to Cart" />
</form>
</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
cart.jsp:
```
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Cart</title>
</head>
<body>
<h1>Cart</h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
<th>Origin</th>
<th>Production Date</th>
<th></th>
</tr>
</thead>
<tbody>
<c:forEach items="${cartItems}" var="item" varStatus="status">
<tr>
<td>${item.name}</td>
<td>${item.price}</td>
<td>${item.origin}</td>
<td>${item.productionDate}</td>
<td>
<form method="post" action="/menu">
<input type="hidden" name="action" value="remove" />
<input type="hidden" name="cartIndex" value="${status.index}" />
<input type="submit" value="Remove" />
</form>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<p>Total: ${total}</p>
<form method="post" action="/menu">
<input type="hidden" name="action" value="clear" />
<input type="submit" value="Clear Cart" />
</form>
</body>
</html>
```
以上就是一个基于MVC设计模式的简单点餐系统的Java Web代码,希望对您有所帮助。
相关推荐
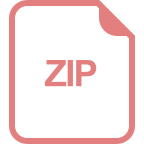
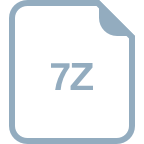
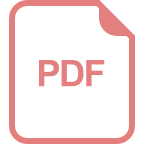














