编写一个C程序实现消息队列 发送两条消息,父进程创建消息队列并发送,子进程接收; 要使用waitpid回收子进程,防止僵尸进程; 消息队列使用完回收消息id(msgctl)
时间: 2024-05-07 12:20:59 浏览: 25
下面是一个实现消息队列的C程序,其中父进程创建并发送两条消息,子进程接收并打印消息内容:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <sys/wait.h>
#define MAX_MSG_LEN 1024
typedef struct {
long type; // 消息类型
char content[MAX_MSG_LEN]; // 消息内容
} message;
int main() {
int msg_id; // 消息队列ID
pid_t pid; // 子进程ID
message msg; // 消息缓冲区
// 创建消息队列
msg_id = msgget(IPC_PRIVATE, 0644);
if (msg_id == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 创建子进程
pid = fork();
if (pid == -1) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid == 0) { // 子进程接收消息
if (msgrcv(msg_id, &msg, MAX_MSG_LEN, 1, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", msg.content);
if (msgrcv(msg_id, &msg, MAX_MSG_LEN, 1, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", msg.content);
// 退出子进程
exit(EXIT_SUCCESS);
} else { // 父进程发送消息
// 发送第一条消息
msg.type = 1;
snprintf(msg.content, MAX_MSG_LEN, "Hello, this is the first message from parent process");
if (msgsnd(msg_id, &msg, strlen(msg.content) + 1, 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
// 发送第二条消息
msg.type = 1;
snprintf(msg.content, MAX_MSG_LEN, "Hello, this is the second message from parent process");
if (msgsnd(msg_id, &msg, strlen(msg.content) + 1, 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
// 等待子进程退出
if (waitpid(pid, NULL, 0) == -1) {
perror("waitpid error");
exit(EXIT_FAILURE);
}
// 删除消息队列
if (msgctl(msg_id, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(EXIT_FAILURE);
}
// 退出父进程
exit(EXIT_SUCCESS);
}
}
```
在程序中,父进程创建消息队列并发送两条消息,子进程接收并打印消息内容。父进程等待子进程退出后,删除消息队列并退出。注意,发送消息时要指定消息类型,接收消息时要指定正确的类型。此外,为防止僵尸进程,需要使用`waitpid`回收子进程。最后,使用`msgctl`删除消息队列。
相关推荐
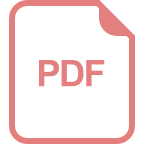
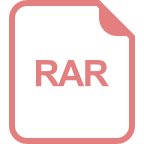
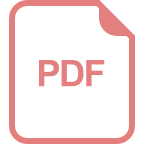















