用C语言实现一个可以用菜单控制,用动态存储实现单链表的创建,单链表的插入,单链表的删除,单链表的查找和单链表的显示,并且可以将顺序表LA和lb的数据元素按非递减有序排列,以及将LA和lb归并为一个新的顺序表Lc而且,Lc中的元素仍然按非递减有序排列
时间: 2023-05-26 11:06:22 浏览: 76
此处提供一个参考实现,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
typedef int DataType;
typedef struct ListNode {
DataType data;
struct ListNode* next;
} ListNode;
ListNode* createList();
ListNode* insertList(ListNode* head, DataType x);
ListNode* deleteList(ListNode* head, DataType x);
ListNode* findList(ListNode* head, DataType x);
void displayList(ListNode* head);
ListNode* mergeList(ListNode* la, ListNode* lb);
void sortList(ListNode* head);
int main() {
ListNode* la = NULL;
ListNode* lb = NULL;
ListNode* lc = NULL;
int choice, x;
do {
printf("\n--- Menu ---\n");
printf("1. Create List\n");
printf("2. Insert Element\n");
printf("3. Delete Element\n");
printf("4. Find Element\n");
printf("5. Display List\n");
printf("6. Merge Lists\n");
printf("7. Sort List\n");
printf("8. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
la = createList();
printf("List Created!\n");
break;
case 2:
printf("Enter the element to insert: ");
scanf("%d", &x);
la = insertList(la, x);
printf("Element Inserted!\n");
break;
case 3:
printf("Enter the element to delete: ");
scanf("%d", &x);
la = deleteList(la, x);
printf("Element Deleted!\n");
break;
case 4:
printf("Enter the element to find: ");
scanf("%d", &x);
ListNode* node = findList(la, x);
if (node == NULL) {
printf("Element not found!\n");
}
else {
printf("Element found at address %p\n", node);
}
break;
case 5:
displayList(la);
break;
case 6:
lb = createList();
lc = mergeList(la, lb);
printf("Lists Merged and Sorted!\n");
printf("LA: ");
displayList(la);
printf("LB: ");
displayList(lb);
printf("LC: ");
displayList(lc);
break;
case 7:
sortList(la);
printf("List Sorted!\n");
break;
case 8:
printf("Goodbye!\n");
break;
default:
printf("Invalid Choice, Try Again!\n");
break;
}
} while (choice != 8);
return 0;
}
ListNode* createList() {
ListNode* head = NULL;
ListNode* tail = NULL;
int n, x;
printf("Enter the number of elements to insert: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter the %d-th element: ", i + 1);
scanf("%d", &x);
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->data = x;
node->next = NULL;
if (head == NULL) {
head = node;
}
else {
tail->next = node;
}
tail = node;
}
return head;
}
ListNode* insertList(ListNode* head, DataType x) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->data = x;
node->next = NULL;
if (head == NULL || x < head->data) {
node->next = head;
head = node;
}
else {
ListNode* p = head;
while (p->next != NULL && x > p->next->data) {
p = p->next;
}
node->next = p->next;
p->next = node;
}
return head;
}
ListNode* deleteList(ListNode* head, DataType x) {
if (head == NULL) {
return NULL;
}
if (head->data == x) {
ListNode* temp = head;
head = head->next;
free(temp);
return head;
}
ListNode* p = head;
while (p->next != NULL && p->next->data != x) {
p = p->next;
}
if (p->next == NULL) {
return head;
}
ListNode* temp = p->next;
p->next = temp->next;
free(temp);
return head;
}
ListNode* findList(ListNode* head, DataType x) {
ListNode* p = head;
while (p != NULL && p->data != x) {
p = p->next;
}
return p;
}
void displayList(ListNode* head) {
ListNode* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
ListNode* mergeList(ListNode* la, ListNode* lb) {
ListNode* lc = NULL;
ListNode* tail = NULL;
while (la != NULL && lb != NULL) {
if (la->data < lb->data) {
if (lc == NULL) {
lc = la;
tail = la;
}
else {
tail->next = la;
tail = la;
}
la = la->next;
}
else {
if (lc == NULL) {
lc = lb;
tail = lb;
}
else {
tail->next = lb;
tail = lb;
}
lb = lb->next;
}
}
if (la != NULL) {
if (lc == NULL) {
lc = la;
}
else {
tail->next = la;
}
}
if (lb != NULL) {
if (lc == NULL) {
lc = lb;
}
else {
tail->next = lb;
}
}
return lc;
}
void sortList(ListNode* head) {
if (head == NULL || head->next == NULL) {
return;
}
ListNode* p = head;
ListNode* q = head->next;
while (q != NULL) {
if (p->data > q->data) {
DataType temp = p->data;
p->data = q->data;
q->data = temp;
}
p = p->next;
q = q->next;
}
sortList(head->next);
}
```
阅读全文
相关推荐
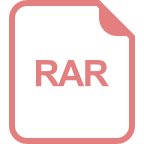

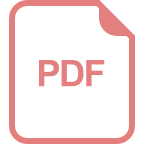
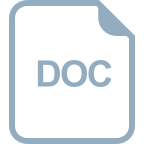




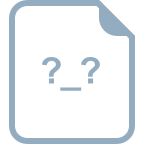
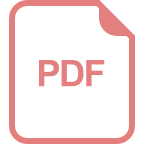
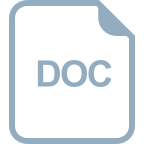
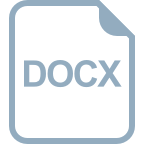
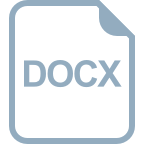
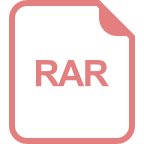
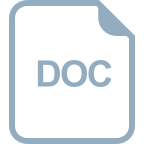
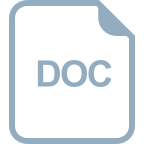