#include<stdio.h> #include<string.h> typedef struct date { int year; int month; int day; } DATE; typedef struct student { int num; char name[20]; DATE date; int score; } STUDENT; void inputarr(STUDENT *b, int n); void maxarr(STUDENT *b, int n); void outputarr(STUDENT *b, int n); int main() { int n; inputarr(b,n); n = maxarr(b, n); outputarr(b, n); printf("第%d个同学的成绩最高。\n", n + 1); return 0; } void inputarr(STUDENT *b, int n) { printf("请输入%d个同学的学号、姓名和成绩:\n", n); for (int i = 0; i < n; i++) { scanf("%d", &b[i].num); getchar(); // 去除输入缓冲区中的回车符 strcpy(b[i].name, ""); DATE d; scanf("%d-%d-%d", &d.year, &d.month, &d.day); b[i].date = d; b[i].score = 0; } } void maxarr(STUDENT *b, int n) { int max_score = 0; int max_num = -1; for (int i = 0; i < n; i++) { if (b[i].score > max_score) { max_score = b[i].score; max_num = i + 1; } } b[max_num].score = max_score; } void outputarr(STUDENT *b, int n) { printf("学号\t姓名\t成绩\n"); for (int i = 0; i < n; i++) { printf("%d\t%s\t%d\n", b[i].num, b[i].name, b[i].score); } }纠错
时间: 2024-04-05 07:35:35 浏览: 85
这是一个 C 语言程序,主要实现了一个学生信息管理系统。程序定义了两个结构体类型:DATE 和 STUDENT。其中 DATE 类型表示日期,包括年、月、日三个成员变量;STUDENT 类型表示学生,包括学号、姓名、出生日期、成绩四个成员变量。程序主要包含三个函数:inputarr、maxarr 和 outputarr。其中 inputarr 函数用于输入学生信息,maxarr 函数用于查找成绩最高的学生并更新其成绩,outputarr 函数用于输出所有学生的信息。程序中存在一些问题,比如定义了指向 STUDENT 类型的指针变量 b,但没有为其分配内存空间;maxarr 函数应该返回成绩最高的学生的下标,而不是成绩最高的学生的成绩。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_STUDENTS 100 // 最多支持100个学生 struct date{ int year; int month; int day; }; typedef struct { int num; // 学号 char name[20]; // 姓名 char sex[5]; // 性别 struct date birthday; // 出生日期 int score[3]; // 三门功课成绩 int total; // 总分 double average; // 平均分 } student; student students[MAX_STUDENTS]; // 学生数组 int num_students = 0; // 学生数量
这段代码定义了一个名为student的结构体,其中包含了学生的学号、姓名、性别、出生日期、三门功课成绩、总分和平均分等信息。同时还定义了一个名为date的结构体,用来表示学生的出生日期。
另外还定义了一个名为students的数组来存储学生信息,数组的长度为MAX_STUDENTS,即最多支持100个学生。还定义了一个变量num_students用来记录当前学生数量。
这段代码的作用是定义了一些数据结构和变量,用来存储和处理学生信息。具体的操作需要在其他代码中实现。
#include<stdio.h> #include<string.h> typedef struct date { int year; int month; int day; }DATE; typedef struct student { int num; char name[20]; char sex; DATE birthday; float score; }STUDENT; void input(STUDENT *s); void output(STUDENT s); /********** Begin **********/ /********** End **********/
void sort(STUDENT s[], int n) {
int i, j;
STUDENT temp;
for (i = 0; i < n - 1; i++) {
for (j = i + 1; j < n; j++) {
if (s[i].score < s[j].score) {
temp = s[i];
s[i] = s[j];
s[j] = temp;
}
}
}
}
在这段代码中,定义了一个sort函数,用于对学生信息按照成绩从高到低排序。函数接受一个学生信息数组s和数组长度n作为参数,使用冒泡排序对s数组进行排序。可以将这段代码放在上述代码之后。
阅读全文
相关推荐
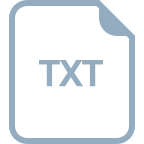
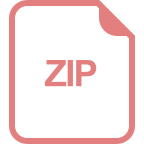
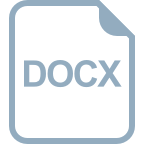




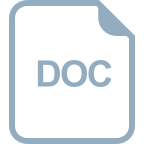
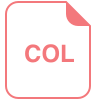






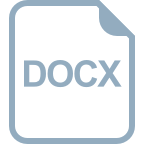