⑹ 编写main函数,声明结构数组a、b并用测试数据初始化(a、b数组中数据按成员id值升序存储;显示a,b数组中数据(显示数组数据调用display函数完成,下同);调delete函数从a数组中删除在b数组中重复出现的联系人信息,显示a数组中数据;调merge函数将a数组中全部联系人信息合并到b数组,显示b数组中数据;调sort函数对a数组中数据排序,显示a数组中数据。
时间: 2023-12-03 22:45:31 浏览: 35
以下是代码实现:
```c++
#include <iostream>
#include <algorithm>
#include "contact.h" // 假设结构体定义在contact.h中
using namespace std;
// 显示联系人信息
void display(Contact *c, int n) {
for (int i = 0; i < n; i++) {
cout << "ID: " << c[i].id << endl;
cout << "Name: " << c[i].name << endl;
cout << "Phone: " << c[i].phone << endl;
cout << "Email: " << c[i].email << endl;
cout << endl;
}
}
// 删除a数组中在b数组中重复出现的联系人信息
// 返回删除后a数组中联系人信息的个数
int deleteRepeat(Contact *a, int n, Contact *b, int m) {
int count = n;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (a[i].id == b[j].id) {
// 在a数组中删除该联系人信息
for (int k = i; k < n - 1; k++) {
a[k] = a[k + 1];
}
i--; // 因为删除了一个元素,所以i要减1
count--; // 删除后联系人信息的个数减1
break;
}
}
}
return count;
}
// 将a数组中的联系人信息合并到b数组中
// 返回合并后b数组中联系人信息的个数
int merge(Contact *a, int n, Contact *b, int m) {
int count = m;
for (int i = 0; i < n; i++) {
b[count++] = a[i];
}
return count;
}
// 按联系人id值升序排序
void sort(Contact *c, int n) {
sort(c, c + n, [](Contact a, Contact b) { return a.id < b.id; });
}
int main() {
// 声明结构数组a、b并用测试数据初始化
Contact a[5] = {{1, "Tom", "123456", "tom@abc.com"},
{2, "Jack", "234567", "jack@abc.com"},
{3, "Mary", "345678", "mary@abc.com"},
{4, "Bob", "456789", "bob@abc.com"},
{5, "Alice", "567890", "alice@abc.com"}};
Contact b[8] = {{1, "Tom", "123456", "tom@abc.com"},
{3, "Mary", "345678", "mary@abc.com"},
{4, "Bob", "456789", "bob@abc.com"},
{6, "John", "678901", "john@abc.com"},
{7, "Lucy", "789012", "lucy@abc.com"},
{8, "David", "890123", "david@abc.com"},
{9, "Sarah", "901234", "sarah@abc.com"},
{10, "Mike", "012345", "mike@abc.com"}};
int n = 5; // a数组中联系人信息的个数
int m = 8; // b数组中联系人信息的个数
// 显示a,b数组中数据
cout << "Array a:" << endl;
display(a, n);
cout << "Array b:" << endl;
display(b, m);
// 调delete函数从a数组中删除在b数组中重复出现的联系人信息,显示a数组中数据
n = deleteRepeat(a, n, b, m);
cout << "After deleting repeat contacts, array a:" << endl;
display(a, n);
// 调merge函数将a数组中全部联系人信息合并到b数组,显示b数组中数据
m = merge(a, n, b, m);
cout << "After merging contacts, array b:" << endl;
display(b, m);
// 调sort函数对a数组中数据排序,显示a数组中数据
sort(a, n);
cout << "After sorting, array a:" << endl;
display(a, n);
return 0;
}
```
相关推荐
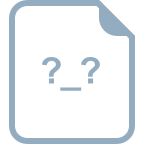
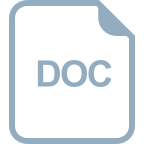
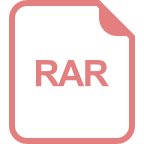














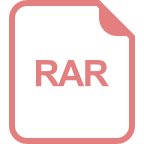