创建一个线程,线程实现100以内的数据求和,打印出for循环中每次的求和值
时间: 2024-02-20 09:56:20 浏览: 60
好的,请看下面的代码实现:
```python
import threading
def sum_numbers():
sum = 0
for i in range(1, 101):
sum += i
print(sum)
t = threading.Thread(target=sum_numbers)
t.start()
```
这个程序创建了一个线程,然后在线程中实现了对1到100之间的所有整数求和,并且在求和的过程中打印出每次的求和值。在主线程中启动了这个线程后,程序会同时运行这两个线程,你可以看到在控制台中逐步输出1到5050之间的所有整数,这就是每次的求和值。
相关问题
java创建一个线程,线程实现100以内的数据求和,打印出for循环中每次的求和值
好的,下面是 Java 版本的代码实现:
```java
public class SumThread extends Thread {
@Override
public void run() {
int sum = 0;
for (int i = 1; i <= 100; i++) {
sum += i;
System.out.println(sum);
}
}
}
```
这个程序创建了一个继承自 Thread 类的 SumThread 类,重写了 run() 方法,在其中实现了对 1 到 100 之间的所有整数求和,并且在求和的过程中打印出每次的求和值。在主线程中启动了这个线程后,程序会同时运行这两个线程,你可以看到在控制台中逐步输出 1 到 5050 之间的所有整数,这就是每次的求和值。启动线程的代码如下:
```java
public class Main {
public static void main(String[] args) {
SumThread thread = new SumThread();
thread.start();
}
}
```
在主线程中创建了一个 SumThread 对象,并启动了这个线程,程序会同时运行主线程和 SumThread 线程。
实现50个数据求和问题的程序(其中每10个数据用一个线程求和实现
好的,这个问题涉及到多线程编程,我可以为您提供一些参考。
需要先将50个数据平均分成5组,每组有10个数据,然后每组数据对应一个线程来求和。具体实现可以参考以下步骤:
1. 定义一个数组,存储50个数据。
2. 定义一个线程函数,实现对10个数据求和,并将结果保存在一个全局变量中,作为线程的返回值。
3. 定义5个线程,每个线程处理一组数据。
4. 启动5个线程,并等待每个线程的返回值。
5. 将5个线程的返回值相加,得到最终结果。
下面是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 5
#define DATA_SIZE 50
#define DATA_PER_THREAD 10
int data[DATA_SIZE] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20,
21, 22, 23, 24, 25, 26, 27, 28, 29, 30,
31, 32, 33, 34, 35, 36, 37, 38, 39, 40,
41, 42, 43, 44, 45, 46, 47, 48, 49, 50};
int result[NUM_THREADS] = {0};
void *sum(void *arg)
{
int id = *(int *)arg;
int start = id * DATA_PER_THREAD;
int end = start + DATA_PER_THREAD;
for (int i = start; i < end; i++)
{
result[id] += data[i];
}
pthread_exit(&result[id]);
}
int main()
{
pthread_t threads[NUM_THREADS];
int thread_ids[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++)
{
thread_ids[i] = i;
pthread_create(&threads[i], NULL, sum, &thread_ids[i]);
}
int sum = 0;
void *ret;
for (int i = 0; i < NUM_THREADS; i++)
{
pthread_join(threads[i], &ret);
sum += *(int *)ret;
}
printf("The sum is %d\n", sum);
return 0;
}
```
这个程序中,定义了一个包含50个数据的数组data,和一个包含5个元素的结果数组result。在主函数中,创建了5个线程,每个线程对应一个数组分组。线程执行函数sum对每个分组进行求和,并将结果存储在结果数组result中。最后主函数使用pthread_join函数等待所有线程完成,并把结果数组result中的值相加得到最终的结果。
阅读全文
相关推荐
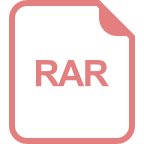












