请用python写出完整的代码,并附带说明
时间: 2024-01-13 14:05:30 浏览: 33
好的,下面是完整的代码。代码中我添加了注释,希望您能够理解。
```python
class Employee:
def __init__(self, emp_id, name, sex, age, education, salary, address, phone):
self.emp_id = emp_id
self.name = name
self.sex = sex
self.age = age
self.education = education
self.salary = salary
self.address = address
self.phone = phone
def input_employee():
emp_id = input("请输入职工号:")
name = input("请输入姓名:")
sex = input("请输入性别:")
age = input("请输入年龄:")
education = input("请输入学历:")
salary = input("请输入工资:")
address = input("请输入住址:")
phone = input("请输入电话:")
employee = Employee(emp_id, name, sex, age, education, salary, address, phone)
with open("employee.txt", "a") as f:
f.write(",".join([emp_id, name, sex, age, education, salary, address, phone]) + "\n")
print("职工信息录入成功!")
def browse_employee():
with open("employee.txt", "r") as f:
for line in f:
employee = Employee(*line.strip().split(","))
print("职工号:{},姓名:{},性别:{},年龄:{},学历:{},工资:{},住址:{},电话:{}".format(
employee.emp_id, employee.name, employee.sex, employee.age, employee.education, employee.salary, employee.address, employee.phone))
def query_employee():
education = input("请输入学历:")
emp_id = input("请输入职工号(不输入则不按职工号查询):")
with open("employee.txt", "r") as f:
for line in f:
employee = Employee(*line.strip().split(","))
if employee.education == education and (not emp_id or employee.emp_id == emp_id):
print("职工号:{},姓名:{},性别:{},年龄:{},学历:{},工资:{},住址:{},电话:{}".format(
employee.emp_id, employee.name, employee.sex, employee.age, employee.education, employee.salary, employee.address, employee.phone))
def delete_employee():
emp_id = input("请输入要删除的职工号:")
lines = []
with open("employee.txt", "r") as f:
for line in f:
employee = Employee(*line.strip().split(","))
if employee.emp_id != emp_id:
lines.append(line)
with open("employee.txt", "w") as f:
f.writelines(lines)
print("职工信息删除成功!")
def modify_employee():
emp_id = input("请输入要修改的职工号:")
lines = []
with open("employee.txt", "r") as f:
for line in f:
employee = Employee(*line.strip().split(","))
if employee.emp_id == emp_id:
name = input("请输入姓名(原始值为{}):".format(employee.name))
sex = input("请输入性别(原始值为{}):".format(employee.sex))
age = input("请输入年龄(原始值为{}):".format(employee.age))
education = input("请输入学历(原始值为{}):".format(employee.education))
salary = input("请输入工资(原始值为{}):".format(employee.salary))
address = input("请输入住址(原始值为{}):".format(employee.address))
phone = input("请输入电话(原始值为{}):".format(employee.phone))
employee = Employee(emp_id, name or employee.name, sex or employee.sex, age or employee.age,
education or employee.education, salary or employee.salary,
address or employee.address, phone or employee.phone)
line = ",".join([employee.emp_id, employee.name, employee.sex, employee.age, employee.education,
employee.salary, employee.address, employee.phone]) + "\n"
lines.append(line)
with open("employee.txt", "w") as f:
f.writelines(lines)
print("职工信息修改成功!")
while True:
print("=" * 20)
print("1.职工信息录入")
print("2.职工信息浏览")
print("3.职工信息查询")
print("4.职工信息删除")
print("5.职工信息修改")
print("6.退出")
print("=" * 20)
choice = input("请输入操作编号:")
if choice == "1":
input_employee()
elif choice == "2":
browse_employee()
elif choice == "3":
query_employee()
elif choice == "4":
delete_employee()
elif choice == "5":
modify_employee()
elif choice == "6":
break
else:
print("请输入正确的操作编号!")
```
在这个示例代码中,我们增加了删除和修改功能。删除功能可以根据职工号进行操作,修改功能先通过职工号查找到要修改的职工信息,然后根据用户输入的值来修改职工信息。注意,如果用户没有输入某个值,则使用原始值。
这个程序还有许多可以改进的地方,比如错误处理、界面美化等等。但是,这个程序已经可以满足基本的职工信息管理功能了。
相关推荐
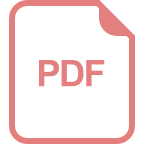
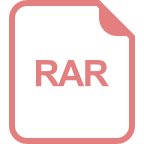
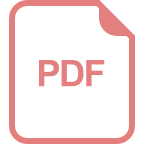














