利用c++,在不利用OpenCv的情况下,如何使用工业相机实现图像灰度化、二值化
时间: 2024-02-19 11:57:55 浏览: 21
在不使用OpenCV的情况下,可以使用基础的C++语言和相机SDK实现图像处理。以下是一个示例代码,展示如何使用MvSDK实现图像灰度化、二值化:
```c++
#include <iostream>
#include "MvCameraControl.h"
using namespace std;
int main()
{
// 打开相机
MV_CC_DEVICE_INFO_LIST deviceList;
MV_CC_GetDeviceList(&deviceList);
if (deviceList.nDeviceNum == 0) {
cout << "无法找到相机!" << endl;
return -1;
}
MV_CC_DEVICE_INFO deviceInfo = deviceList.pDeviceInfo[0];
void* handle;
MV_CC_OpenDevice(deviceInfo.nTLayerType, deviceInfo.pszProductSeries, deviceInfo.pszAddr, deviceInfo.nPort, &handle);
// 调整相机参数
MVCC_INTVALUE stIntValue;
MV_CC_GetIntValue(handle, "Width", &stIntValue);
stIntValue.nCurValue = 640;
MV_CC_SetIntValue(handle, "Width", stIntValue.nCurValue);
MV_CC_GetIntValue(handle, "Height", &stIntValue);
stIntValue.nCurValue = 480;
MV_CC_SetIntValue(handle, "Height", stIntValue.nCurValue);
// 开始取图
MV_CC_StartGrabbing(handle);
while (true) {
// 获取一帧图像
MV_FRAME_OUT frame;
MV_CC_GetImageForBGR(handle, &frame, 1000);
// 灰度化
unsigned char* grayData = new unsigned char[frame.nFrameLen];
for (int i = 0; i < frame.nFrameLen; i += 3) {
grayData[i / 3] = (unsigned char)(0.299 * (double)frame.pBuf[i + 2] + 0.587 * (double)frame.pBuf[i + 1] + 0.114 * (double)frame.pBuf[i]);
}
// 二值化
unsigned char* binaryData = new unsigned char[frame.nFrameLen / 3];
int thresholdValue = 128;
for (int i = 0; i < frame.nFrameLen / 3; i++) {
binaryData[i] = grayData[i] > thresholdValue ? 255 : 0;
}
// 显示图像
cv::Mat mat(frame.nHeight, frame.nWidth, CV_8UC3, frame.pBuf);
cv::imshow("Camera", mat);
cv::Mat grayMat(frame.nHeight, frame.nWidth, CV_8UC1, grayData);
cv::imshow("Gray", grayMat);
cv::Mat binaryMat(frame.nHeight, frame.nWidth, CV_8UC1, binaryData);
cv::imshow("Binary", binaryMat);
// 释放内存
delete[] grayData;
delete[] binaryData;
// 等待按键
if (cv::waitKey(30) == 27) {
break;
}
}
// 停止取图
MV_CC_StopGrabbing(handle);
// 关闭相机
MV_CC_CloseDevice(handle);
return 0;
}
```
在上述代码中,使用MvSDK打开相机,并设置相机的分辨率。然后循环读取相机的每一帧图像,并进行灰度化、二值化处理。最后使用cv::imshow显示处理过的图像,并使用cv::waitKey等待按键。需要注意的是,灰度化和二值化的处理使用了基础的像素操作,没有使用OpenCV函数。
相关推荐
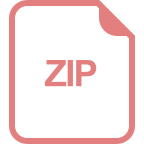
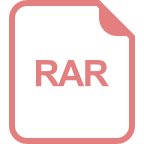














