遗传算法的python实现
时间: 2023-12-04 10:30:50 浏览: 29
遗传算法是一种基于生物进化理论的优化算法,它通过基因交叉、变异、选择等操作,模拟自然界中的遗传进化过程,实现对问题的求解。下面是一个简单的遗传算法的Python实现示例:
```python
import random
# 目标函数(示例为简单的函数 f(x) = x^2)
def fitness(x):
return x**2
# 个体类
class Individual:
def __init__(self, x):
self.x = x
self.fitness = fitness(x)
# 种群类
class Population:
def __init__(self, size):
self.size = size
self.individuals = []
for i in range(size):
x = random.uniform(-10, 10) # 随机生成初始个体
individual = Individual(x)
self.individuals.append(individual)
# 选择操作(轮盘赌选择)
def selection(self):
total_fitness = sum([ind.fitness for ind in self.individuals])
r = random.uniform(0, total_fitness)
s = 0
for ind in self.individuals:
s += ind.fitness
if s >= r:
return ind
# 交叉操作(单点交叉)
def crossover(self, ind1, ind2):
x1 = ind1.x
x2 = ind2.x
p = random.uniform(0, 1)
x3 = p * x1 + (1 - p) * x2
x4 = (1 - p) * x1 + p * x2
ind3 = Individual(x3)
ind4 = Individual(x4)
return ind3, ind4
# 变异操作(高斯变异)
def mutation(self, ind, mutation_rate):
x = ind.x
if random.uniform(0, 1) < mutation_rate:
x = x + random.gauss(0, 1)
return Individual(x)
# 进化过程
def evolve(self, pc, pm):
new_individuals = []
for i in range(self.size//2):
# 选择两个个体
ind1 = self.selection()
ind2 = self.selection()
# 交叉
if random.uniform(0, 1) < pc:
ind3, ind4 = self.crossover(ind1, ind2)
else:
ind3, ind4 = ind1, ind2
# 变异
ind3 = self.mutation(ind3, pm)
ind4 = self.mutation(ind4, pm)
# 加入新种群
new_individuals.append(ind3)
new_individuals.append(ind4)
self.individuals = new_individuals
if __name__ == '__main__':
# 初始化种群
pop = Population(10)
# 迭代100次
for i in range(100):
# 进化种群
pop.evolve(0.8, 0.2)
# 打印最优个体
best_ind = max(pop.individuals, key=lambda ind: ind.fitness)
print('Generation {}: x={}, fitness={}'.format(i+1, best_ind.x, best_ind.fitness))
```
该实现中,个体类`Individual`包含一个基因`x`和一个适应度`fitness`;种群类`Population`包含一个个体列表`individuals`,以及选择、交叉、变异、进化等操作方法。在主函数中,我们初始化一个种群并进行100代进化,每次迭代后打印最优个体的信息。
相关推荐
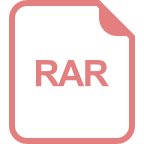
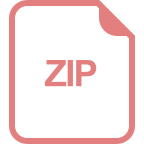
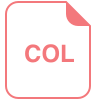
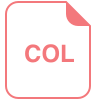
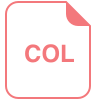
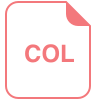
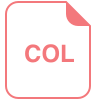









