这是一个猜词游戏的规则说明,需要玩家或电脑根据设定的条件和提示来猜测正确的单词或组合。玩法包括猜单词和猜组合两种,猜单词需要猜出单词中的字母,猜组合需要猜出电脑设定的模式。游戏中有猜错次数限制和重复猜测限制,需要玩家或电脑在规定次数内猜出正确答案。每次猜测后,游戏都会显示当前猜出的残缺单词或猜测的组合情况,以及已经猜错的字母和剩余猜错次数。游戏的目标是在规定次数内猜出正确的单词或组合。添加是否在玩一次功能.用c++写出并表明思路
时间: 2023-12-06 08:45:11 浏览: 139
思路:
1. 定义一个单词库,将要猜的单词或组合存储其中。
2. 确定猜错次数和重复猜测限制,可以使用计数器来实现。
3. 对于猜单词的模式,需要将单词中每个字母用下划线代替,每次猜测后将正确猜出的字母替换相应的下划线。
4. 对于猜组合的模式,需要定义一定的模式规则,并将其存储在程序中。每次猜测后将符合规则的组合显示出来。
5. 在每次猜测后,需要将已经猜错的字母记录下来,并在剩余猜错次数减少时显示出来。
6. 当剩余猜错次数为0或者猜测正确时,游戏结束。
7. 可以添加是否继续玩一次的功能。
代码实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
vector<string> words = {"apple", "banana", "orange", "watermelon", "strawberry", "pear", "peach", "grape", "pineapple", "kiwi"};
void play_game()
{
srand(time(NULL));
int random_index = rand() % words.size();
string word_to_guess = words[random_index];
int incorrect_guesses = 0;
int max_guesses = 5;
string guessed_word(word_to_guess.length(), '_');
vector<char> incorrect_letters;
while (incorrect_guesses < max_guesses && guessed_word != word_to_guess)
{
cout << "Guess the word: " << guessed_word << endl;
cout << "You have guessed the following incorrect letters: ";
for (char letter : incorrect_letters)
{
cout << letter << " ";
}
cout << endl;
char guess;
cout << "Enter your guess: ";
cin >> guess;
if (find(incorrect_letters.begin(), incorrect_letters.end(), guess) != incorrect_letters.end())
{
cout << "You already guessed that letter. Try again." << endl;
continue;
}
bool incorrect_guess = true;
for (int i = 0; i < word_to_guess.length(); i++)
{
if (word_to_guess[i] == guess)
{
guessed_word[i] = guess;
incorrect_guess = false;
}
}
if (incorrect_guess)
{
incorrect_guesses++;
incorrect_letters.push_back(guess);
cout << "Incorrect guess. You have " << max_guesses - incorrect_guesses << " guesses left." << endl;
}
}
if (incorrect_guesses == max_guesses)
{
cout << "You lost! The word was " << word_to_guess << "." << endl;
}
else
{
cout << "Congratulations! You guessed the word " << word_to_guess << "!" << endl;
}
}
int main()
{
char play_again = 'y';
while (play_again == 'y')
{
play_game();
cout << "Do you want to play again? (y/n) ";
cin >> play_again;
}
return 0;
}
```
以上是猜单词游戏的实现代码,其中使用了随机数生成器来随机选择要猜的单词,使用了vector来存储已经猜错的字母。玩家每次猜测后,程序会判断是否猜对了,如果猜对了就会将正确的字母替换相应的下划线,如果猜错了就会将猜错的字母记录下来,并在剩余猜错次数减少时显示出来。游戏结束时,会显示出猜测结果。最后,程序会询问玩家是否继续玩一次,如果玩家选择继续,则重新开始游戏,否则程序结束。
阅读全文
相关推荐
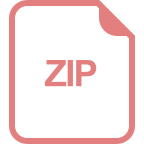

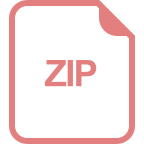
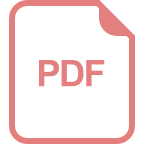
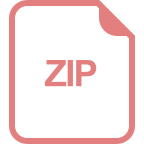
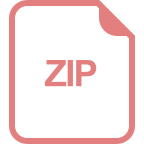
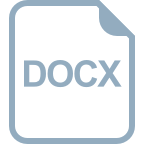
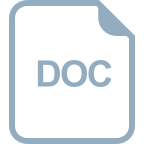
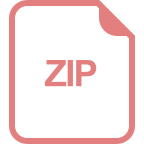
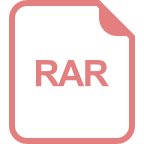
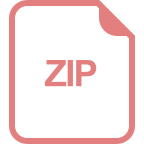
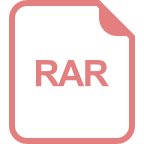
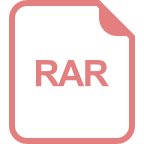
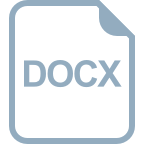