Java中如何设置Excel表格列宽
时间: 2023-05-17 08:03:17 浏览: 624
您可以使用POI库中的setColumnWidth方法来设置Excel表格列宽。具体代码如下:
```
// 创建工作簿
Workbook workbook = new XSSFWorkbook();
// 创建工作表
Sheet sheet = workbook.createSheet("Sheet1");
// 设置第一列宽度为20个字符
sheet.setColumnWidth(0, 20 * 256);
```
其中,第一个参数为列的索引,第二个参数为列宽,单位为1/256个字符。
相关问题
java如何设置Excel表格中第二行,第二列的文字水平居中
您可以使用 Apache POI 库来设置 Excel 表格中的文字水平居中。以下是示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelDemo {
public static void main(String[] args) throws IOException {
// 创建工作簿
Workbook workbook = WorkbookFactory.create(true);
// 创建工作表
Sheet sheet = workbook.createSheet("Sheet1");
// 创建行
Row row = sheet.createRow(1);
// 创建单元格
Cell cell = row.createCell(1);
// 设置单元格样式
CellStyle style = workbook.createCellStyle();
style.setAlignment(HorizontalAlignment.CENTER); // 设置水平居中
// 设置单元格内容
cell.setCellValue("Hello, World!");
// 应用样式
cell.setCellStyle(style);
// 保存工作簿
FileOutputStream outputStream = new FileOutputStream("demo.xlsx");
workbook.write(outputStream);
outputStream.close();
}
}
```
这段代码将在 Excel 表格中创建一个单元格,将其内容设置为 "Hello, World!",并将其水平居中。
Java中如何设置Excel表格某一列的文字水平居中
您可以使用Apache POI库来操作Excel表格。以下是一个示例代码片段,可以将Excel表格中的某一列的文字水平居中:
```
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.*;
public class ExcelUtils {
public static void setColumnTextHorizontalAlignment(File file, String sheetName, int columnIndex) throws IOException {
Workbook workbook = new XSSFWorkbook(new FileInputStream(file));
Sheet sheet = workbook.getSheet(sheetName);
CellStyle style = workbook.createCellStyle();
style.setAlignment(HorizontalAlignment.CENTER);
for (Row row : sheet) {
Cell cell = row.getCell(columnIndex);
if (cell != null) {
cell.setCellStyle(style);
}
}
FileOutputStream outputStream = new FileOutputStream(file);
workbook.write(outputStream);
workbook.close();
outputStream.close();
}
}
```
您可以将此代码片段添加到您的Java项目中,并调用`setColumnTextHorizontalAlignment`方法,传递Excel文件、工作表名称和要居中的列索引作为参数。
阅读全文
相关推荐
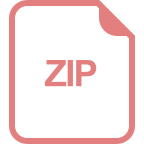
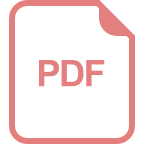
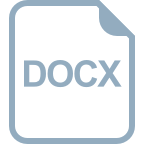
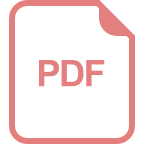
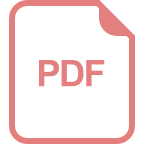
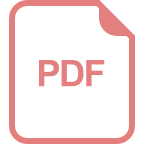
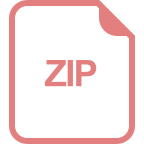
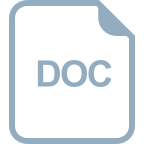
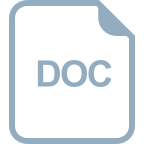
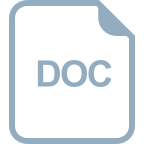
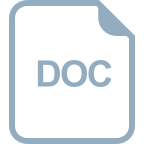



