用C语言实现通过SecrueCRT向开发板发送1,LED灯亮并返回字符串”led on”;发送0,LED灯灭返回字符串”led off”,其他返回”other”。
时间: 2023-06-03 12:06:38 浏览: 71
这个问题是程序设计问题,可以回答。以下是实现方式:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <winsock2.h>
void sendCommand(const char* command, const char* expectedResponse)
{
// 创建Socket
WSADATA wsaData;
WSAStartup(MAKEWORD(2, 2), &wsaData);
SOCKET sock = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP);
struct sockaddr_in sockAddr;
sockAddr.sin_family = AF_INET;
sockAddr.sin_addr.S_un.S_addr = inet_addr("192.168.1.1"); // 修改为开发板的IP地址
sockAddr.sin_port = htons(23); // SecureCRT的端口号为23
connect(sock, (struct sockaddr *)&sockAddr, sizeof(SOCKADDR_IN));
// 发送命令
char buffer[1024] = {0};
send(sock, command, strlen(command), 0);
recv(sock, buffer, 1024, 0);
if (strcmp(buffer, expectedResponse) != 0) {
printf("Error: Expected response %s, but got %s\n", expectedResponse, buffer);
exit(1);
}
closesocket(sock);
WSACleanup();
}
int main()
{
int ledStatus = -1;
char command[10];
char expectedResponse[10];
while (1) {
printf("Enter command (0 for LED off, 1 for LED on): ");
scanf("%d", &ledStatus);
if (ledStatus == 0) {
strcpy(command, "0");
strcpy(expectedResponse, "led off");
} else if (ledStatus == 1) {
strcpy(command, "1");
strcpy(expectedResponse, "led on");
} else {
strcpy(command, "other");
strcpy(expectedResponse, "other");
}
sendCommand(command, expectedResponse);
}
return 0;
}
阅读全文
相关推荐
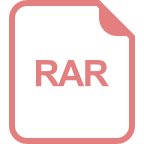
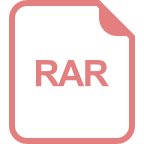

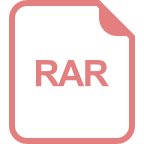
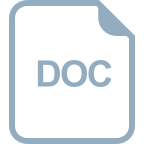








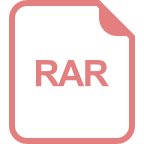
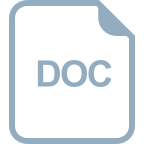


