利用python写一个根据字典替换txt文件中的关键词,并用代码展示
时间: 2024-05-07 22:18:38 浏览: 6
下面是一个例子,假设我们有一个名为`example.txt`的文本文件,我们想要将其中的一些关键词替换为字典中对应的值。
首先,我们需要定义我们的字典,例如:
```python
replacement_dict = {
"cat": "dog",
"apple": "orange",
"happy": "sad"
}
```
然后,我们需要打开我们的文本文件,并读取其中的内容:
```python
with open("example.txt", "r") as file:
text = file.read()
```
接下来,我们需要循环遍历字典中的每一个关键词,并使用Python的字符串替换功能将它们替换为对应的值:
```python
for key, value in replacement_dict.items():
text = text.replace(key, value)
```
最后,我们将替换后的文本写回到文件中:
```python
with open("example.txt", "w") as file:
file.write(text)
```
完整的代码如下:
```python
replacement_dict = {
"cat": "dog",
"apple": "orange",
"happy": "sad"
}
with open("example.txt", "r") as file:
text = file.read()
for key, value in replacement_dict.items():
text = text.replace(key, value)
with open("example.txt", "w") as file:
file.write(text)
```
请注意,这只是一个简单的示例,如果您的文本文件非常大,这种方法可能会变得很慢。在这种情况下,您可能需要使用更高效的算法或库来进行替换。
相关推荐
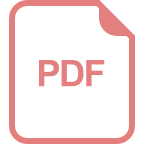













