用C++字典树进行字符串匹配
时间: 2024-04-29 10:23:58 浏览: 69
C语言实现字典树字符串匹配的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NODE 100000 // 最大节点数
#define MAX_WORD 1000 // 最大单词长度
struct TrieNode {
int count; // 记录单词出现的次数
struct TrieNode *child[26]; // 每个节点的子节点,每个子节点代表一个字母
} *root;
// 创建一个新的节点
struct TrieNode *create_node() {
struct TrieNode *node = (struct TrieNode *) malloc(sizeof(struct TrieNode));
node->count = 0;
memset(node->child, 0, sizeof(node->child)); // 将子节点全部初始化为NULL
return node;
}
// 将单词插入到字典树中
void insert(char *word) {
struct TrieNode *node = root;
for (int i = 0; i < strlen(word); i++) {
int index = word[i] - 'a';
if (!node->child[index]) {
node->child[index] = create_node();
}
node = node->child[index];
}
node->count++;
}
// 在字典树中查找单词
int search(char *word) {
struct TrieNode *node = root;
for (int i = 0; i < strlen(word); i++) {
int index = word[i] - 'a';
if (!node->child[index]) {
return 0; // 字典树中不存在该单词
}
node = node->child[index];
}
return node->count; // 返回单词出现的次数
}
int main() {
root = create_node(); // 创建根节点
int n;
char word[MAX_WORD];
printf("请输入单词个数:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("请输入第%d个单词:", i + 1);
scanf("%s", word);
insert(word); // 将单词插入到字典树中
}
printf("请输入要查找的单词:");
scanf("%s", word);
int count = search(word); // 在字典树中查找单词
if (count > 0) {
printf("单词 %s 出现了 %d 次\n", word, count);
} else {
printf("单词 %s 不存在\n", word);
}
return 0;
}
```
使用方法:输入单词个数,依次输入每个单词,然后输入要查找的单词即可。
示例输入输出:
```
请输入单词个数:4
请输入第1个单词:apple
请输入第2个单词:banana
请输入第3个单词:orange
请输入第4个单词:pear
请输入要查找的单词:apple
单词 apple 出现了 1 次
```
阅读全文
相关推荐
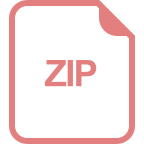

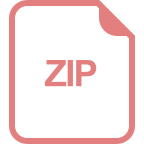
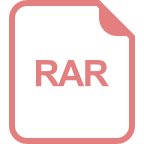
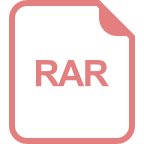
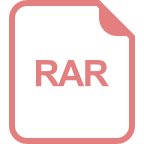
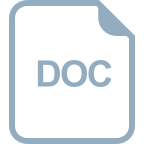
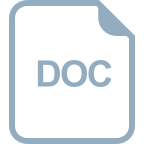
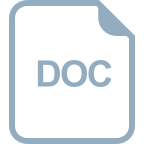
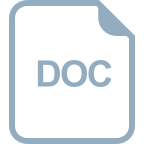
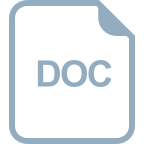


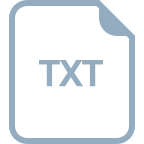
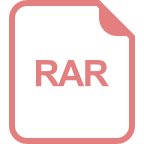
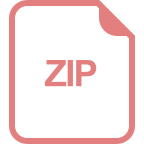