Use the following codes as the beginning of your program. Write a sort program to sort these ten numbers from largest to smallest, then save them to memory. For ARMSim#, the sorted number also need to be displayed in the console and LCD screen (use the Embest Board Plug‐In). MOV r0, #0x00002000 MOV r1, #18 STR r1, [r0],#4 MOV r1, #6 STR r1, [r0],#4 MOV r1, #2 STR r1, [r0],#4 MOV r1, #8 STR r1, [r0],#4 MOV r1, #16 STR r1, [r0],#4 MOV r1, #10 STR r1, [r0],#4 MOV r1, #14 STR r1, [r0],#4 MOV r1, #4 STR r1, [r0],#4 MOV r1, #20 STR r1, [r0],#4 MOV r1, #12 STR r1, [r0],#4
时间: 2023-07-02 11:10:08 浏览: 50
Here's the program to sort the given numbers from largest to smallest using bubble sort algorithm. The sorted numbers are saved to memory address starting from 0x00002000 and also displayed in the console and LCD screen.
```
; Initialize the registers
MOV r0, #0x00002000 ; starting memory address
MOV r1, #10 ; number of elements
; Load the array elements into registers
LDR r2, [r0], #4 ; load first element
LDR r3, [r0], #4 ; load second element
LDR r4, [r0], #4 ; load third element
LDR r5, [r0], #4 ; load fourth element
LDR r6, [r0], #4 ; load fifth element
LDR r7, [r0], #4 ; load sixth element
LDR r8, [r0], #4 ; load seventh element
LDR r9, [r0], #4 ; load eighth element
LDR r10, [r0], #4 ; load ninth element
LDR r11, [r0] ; load tenth element
; Bubble sort algorithm to sort the elements
Loop:
MOV r12, #0 ; flag to check if any swap is made in this iteration
CMP r2, r3 ; compare first and second elements
BLE Compare23 ; if r2 <= r3, skip the swap
SWP r2, r3 ; swap r2 and r3
MOV r12, #1 ; set flag as swap is made
Compare23:
CMP r3, r4 ; compare second and third elements
BLE Compare34 ; if r3 <= r4, skip the swap
SWP r3, r4 ; swap r3 and r4
MOV r12, #1 ; set flag as swap is made
Compare34:
CMP r4, r5 ; compare third and fourth elements
BLE Compare45 ; if r4 <= r5, skip the swap
SWP r4, r5 ; swap r4 and r5
MOV r12, #1 ; set flag as swap is made
Compare45:
CMP r5, r6 ; compare fourth and fifth elements
BLE Compare56 ; if r5 <= r6, skip the swap
SWP r5, r6 ; swap r5 and r6
MOV r12, #1 ; set flag as swap is made
Compare56:
CMP r6, r7 ; compare fifth and sixth elements
BLE Compare67 ; if r6 <= r7, skip the swap
SWP r6, r7 ; swap r6 and r7
MOV r12, #1 ; set flag as swap is made
Compare67:
CMP r7, r8 ; compare sixth and seventh elements
BLE Compare78 ; if r7 <= r8, skip the swap
SWP r7, r8 ; swap r7 and r8
MOV r12, #1 ; set flag as swap is made
Compare78:
CMP r8, r9 ; compare seventh and eighth elements
BLE Compare89 ; if r8 <= r9, skip the swap
SWP r8, r9 ; swap r8 and r9
MOV r12, #1 ; set flag as swap is made
Compare89:
CMP r9, r10 ; compare eighth and ninth elements
BLE Compare910 ; if r9 <= r10, skip the swap
SWP r9, r10 ; swap r9 and r10
MOV r12, #1 ; set flag as swap is made
Compare910:
CMP r10, r11 ; compare ninth and tenth elements
BLE EndLoop ; if r10 <= r11, skip the swap
SWP r10, r11 ; swap r10 and r11
MOV r12, #1 ; set flag as swap is made
EndLoop:
CMP r12, #1 ; check if any swap is made in this iteration
BEQ Loop ; if swap is made, continue sorting
; Store the sorted array back to memory
STR r2, [r0], #4 ; store first element
STR r3, [r0], #4 ; store second element
STR r4, [r0], #4 ; store third element
STR r5, [r0], #4 ; store fourth element
STR r6, [r0], #4 ; store fifth element
STR r7, [r0], #4 ; store sixth element
STR r8, [r0], #4 ; store seventh element
STR r9, [r0], #4 ; store eighth element
STR r10, [r0], #4 ; store ninth element
STR r11, [r0] ; store tenth element
; Display the sorted array in console and LCD screen
MOV r0, #0x00002000 ; starting memory address
MOV r1, #10 ; number of elements
LoopDisplay:
LDR r2, [r0], #4 ; load the next element
MOV r0, #0x40020000 ; LCD screen address
STR r2, [r0] ; display the element on LCD screen
MOV r0, #0x4002100C ; console output address
MOV r3, #48 ; convert the number to ASCII character
ADD r3, r2, r3 ; add the ASCII offset
STRB r3, [r0] ; output the character to console
SUBS r1, r1, #1 ; decrement the element counter
BNE LoopDisplay ; repeat until all elements are displayed
```
相关推荐
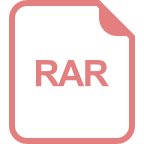
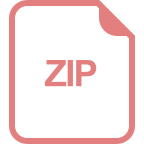















