esp32 python hello world
时间: 2023-07-06 09:32:59 浏览: 40
好的,让我们一起来编写一个ESP32上的Python程序,输出"Hello, world!"。
首先,你需要安装一个串口调试工具,比如PuTTY。通过串口连接ESP32,然后输入以下命令:
```
import time
print("Hello, world!")
```
这段代码会在ESP32上运行,并输出"Hello, world!"。
如果你使用的是MicroPython固件,你也可以通过REPL(交互式解释器)来运行这段代码。在连接上ESP32的串口终端中,输入以下命令:
```
>>> import time
>>> print("Hello, world!")
```
同样,这段代码也会输出"Hello, world!"。
希望这能帮助到你。
相关问题
esp8266-idf的helloworld环境搭
为搭建ESP8266-IDF的helloworld环境,需要进行以下步骤:
1. 安装Ubuntu系统,并安装所需的基本软件,如Python、Git等。
2. 下载ESP8266-IDF源代码,并将其放置在合适的目录下。
3. 配置ESP8266编译环境,包括设置环境变量、安装交叉编译工具链等。
4. 创建一个新的ESP8266项目,并编写helloworld程序。
5. 编译并烧录程序到ESP8266开发板上。
下面是具体的步骤:
1. 安装Ubuntu系统,并安装所需的基本软件,如Python、Git等。可以使用以下命令安装所需软件:
```shell
sudo apt-get update
sudo apt-get install git wget make libncurses-dev flex bison gperf python python-serial
```
2. 下载ESP8266-IDF源代码,并将其放置在合适的目录下。可以使用以下命令下载ESP8266-IDF源代码:
```shell
git clone --recursive https://github.com/espressif/esp8266-idf.git
```
3. 配置ESP8266编译环境,包括设置环境变量、安装交叉编译工具链等。可以使用以下命令配置ESP8266编译环境:
```shell
cd esp8266-idf
./install.sh
```
4. 创建一个新的ESP8266项目,并编写helloworld程序。可以使用以下命令创建一个新的ESP8266项目:
```shell
cd ~/esp8266
cp -r $IDF_PATH/examples/get-started/hello_world .
cd hello_world
```
然后,可以使用任何文本编辑器编写helloworld程序,例如:
```c
#include <stdio.h>
void app_main()
{
printf("Hello, world!\n");
}
```
5. 编译并烧录程序到ESP8266开发板上。可以使用以下命令编译并烧录程序:
```shell
make menuconfig # 配置编译选项
make all # 编译程序
make flash # 烧录程序到ESP8266开发板
```
至此,ESP8266-IDF的helloworld环境搭建完成。
ESP32S2 USB串口通讯 micpython程序
以下是一个基于MicroPython的ESP32S2 USB串口通信示例代码:
```
import machine
import time
uart = machine.UART(0, baudrate=115200) # 初始化USB串口通信,设置波特率为115200
while True:
uart.write('Hello, world!\n') # 向USB串口发送数据
time.sleep(1)
if uart.any(): # 接收从电脑发送的数据
recv_data = uart.read()
print('Received: ', recv_data.decode())
```
在电脑上打开串口监视器,选择与ESP32S2连接的USB串口,并设置波特率为115200。您应该可以看到ESP32S2向串口监视器发送“Hello, world!”,并且可以通过串口监视器向ESP32S2发送数据。
请注意,MicroPython的代码与Arduino IDE的代码略有不同,但逻辑相同。上面的代码也可以通过Arduino IDE进行编写和运行,只需要稍作修改即可。
相关推荐
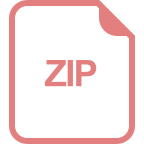












