用Java实现下面的要求:布局计算器:1、在顶部的显示标签,将用来显示计算结果/2、一个文本字段,供用户在中间输入整数或者二倍的文本字段3、在底部有“clear”,“+”,“-”,“*”和“/”的按钮。创建一个累加器状态变量,用以跟踪计算的当前总值。1、点击“清除”按钮,将累加器值设置为0/2、点击“+”按钮,将文本字段值添加到累加器值/3、点击“-”按钮,从累加器值中减去文本字段值/4、点击“*”按钮,将累加段值乘以/5、点击“/”按钮,将累加器值除以文本字段值6、按下CLR按钮后,用新的累加器值更新显示标签,清空文本字段。
时间: 2023-12-25 22:06:38 浏览: 113
以下是用Java实现布局计算器的代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Calculator extends JFrame implements ActionListener {
private JLabel resultLabel;
private JTextField inputField;
private JButton clearButton;
private JButton addButton;
private JButton minusButton;
private JButton multiplyButton;
private JButton divideButton;
private int accumulator;
public Calculator() {
setTitle("Calculator");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
// 创建顶部的显示标签
resultLabel = new JLabel("0", JLabel.RIGHT);
resultLabel.setFont(new Font("Arial", Font.PLAIN, 20));
add(resultLabel, BorderLayout.NORTH);
// 创建中间的文本字段
inputField = new JTextField();
inputField.setFont(new Font("Arial", Font.PLAIN, 20));
add(inputField, BorderLayout.CENTER);
// 创建底部的按钮
JPanel buttonPanel = new JPanel(new GridLayout(1, 5, 5, 5));
clearButton = new JButton("CLR");
addButton = new JButton("+");
minusButton = new JButton("-");
multiplyButton = new JButton("*");
divideButton = new JButton("/");
clearButton.addActionListener(this);
addButton.addActionListener(this);
minusButton.addActionListener(this);
multiplyButton.addActionListener(this);
divideButton.addActionListener(this);
buttonPanel.add(clearButton);
buttonPanel.add(addButton);
buttonPanel.add(minusButton);
buttonPanel.add(multiplyButton);
buttonPanel.add(divideButton);
add(buttonPanel, BorderLayout.SOUTH);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clearButton) {
accumulator = 0;
resultLabel.setText("0");
inputField.setText("");
} else {
int value;
try {
value = Integer.parseInt(inputField.getText());
} catch (NumberFormatException ex) {
value = 0;
}
if (e.getSource() == addButton) {
accumulator += value;
} else if (e.getSource() == minusButton) {
accumulator -= value;
} else if (e.getSource() == multiplyButton) {
accumulator *= value;
} else if (e.getSource() == divideButton) {
if (value == 0) {
JOptionPane.showMessageDialog(this, "除数不能为0!", "错误", JOptionPane.ERROR_MESSAGE);
return;
}
accumulator /= value;
}
resultLabel.setText(String.valueOf(accumulator));
inputField.setText("");
}
}
public static void main(String[] args) {
new Calculator();
}
}
```
运行该程序后,即可看到一个简单的布局计算器界面。用户可以在中间的文本字段中输入整数或者二进制数,然后点击底部的“+”、“-”、“*”、“/”按钮进行计算。点击“CLR”按钮可以清空输入和计算结果。
阅读全文
相关推荐
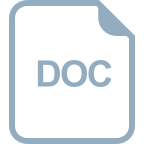
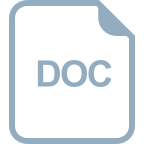
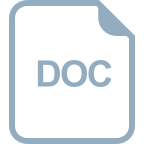


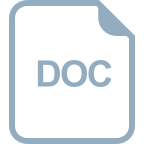
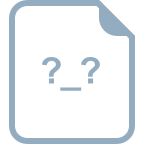
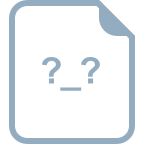
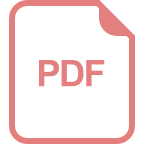
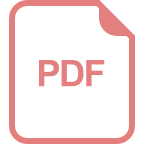
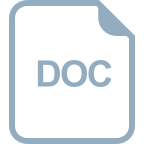
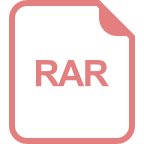
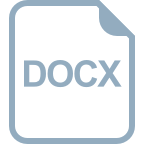
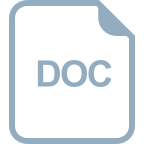
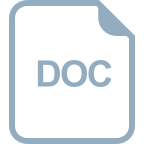
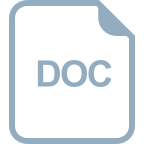
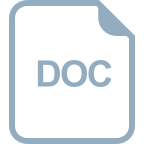
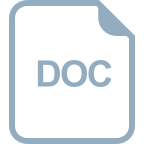
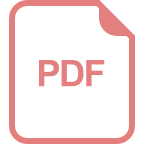
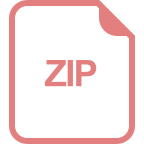