用Java编程:计算器,使用恰当的组件和容器,加完成软件界面设计。
时间: 2024-03-06 18:48:59 浏览: 18
好的,以下是一个简单的Java计算器程序的界面设计和代码实现:
界面设计:
```java
import java.awt.BorderLayout;
import java.awt.GridLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class CalculatorUI {
private JFrame frame;
private JTextField textField;
private JPanel buttonPanel;
public CalculatorUI() {
frame = new JFrame("Calculator");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
textField = new JTextField();
textField.setHorizontalAlignment(JTextField.RIGHT);
frame.add(textField, BorderLayout.NORTH);
buttonPanel = new JPanel(new GridLayout(4, 4));
frame.add(buttonPanel, BorderLayout.CENTER);
addButton("7");
addButton("8");
addButton("9");
addButton("/");
addButton("4");
addButton("5");
addButton("6");
addButton("*");
addButton("1");
addButton("2");
addButton("3");
addButton("-");
addButton("0");
addButton(".");
addButton("=");
addButton("+");
frame.pack();
frame.setVisible(true);
}
private void addButton(String label) {
JButton button = new JButton(label);
button.addActionListener(new CalculatorActionListener(textField, label));
buttonPanel.add(button);
}
public static void main(String[] args) {
new CalculatorUI();
}
}
```
代码实现:
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JTextField;
public class CalculatorActionListener implements ActionListener {
private JTextField textField;
private String label;
private double result = 0;
private String operator = "";
private boolean start = true;
public CalculatorActionListener(JTextField textField, String label) {
this.textField = textField;
this.label = label;
}
public void actionPerformed(ActionEvent e) {
String input = label;
if (input.equals("C")) {
result = 0;
operator = "";
start = true;
textField.setText("");
} else if (input.equals("=")) {
if (operator.equals("+")) {
result += Double.parseDouble(textField.getText());
} else if (operator.equals("-")) {
result -= Double.parseDouble(textField.getText());
} else if (operator.equals("*")) {
result *= Double.parseDouble(textField.getText());
} else if (operator.equals("/")) {
result /= Double.parseDouble(textField.getText());
}
textField.setText("" + result);
operator = "";
start = true;
} else if (input.equals("+") || input.equals("-") || input.equals("*") || input.equals("/")) {
if (start) {
if (input.equals("-")) {
textField.setText(input);
start = false;
} else {
operator = input;
}
} else {
double x = Double.parseDouble(textField.getText());
if (operator.equals("+")) {
result += x;
} else if (operator.equals("-")) {
result -= x;
} else if (operator.equals("*")) {
result *= x;
} else if (operator.equals("/")) {
result /= x;
}
operator = input;
textField.setText("" + result);
}
start = false;
} else {
if (start) {
textField.setText("");
start = false;
}
textField.setText(textField.getText() + input);
}
}
}
```
这个简单的Java计算器程序可以进行加、减、乘、除四则运算,也支持多次连续计算。
相关推荐
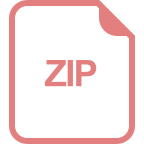
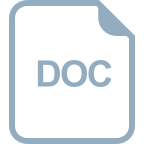
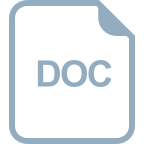














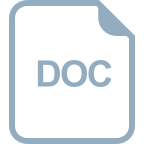