python groupby count
时间: 2023-04-29 20:00:11 浏览: 414
在 Python 中使用 groupby 函数和 count 方法可以对数据进行分组并统计每组数据的个数。
使用方法:
```
from itertools import groupby
data = [1, 2, 2, 3, 3, 3]
#使用 groupby 函数对数据进行分组
grouped_data = groupby(data)
#使用 count 方法统计每组数据的个数
result = {key: len(list(group)) for key, group in grouped_data}
print(result)
#输出: {1: 1, 2: 2, 3: 3}
```
请注意,groupby 函数需要先排序。
如果你使用pandas,可以这样做:
```
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 2, 3, 3, 3]})
result = df.groupby('A').size().reset_index(name='counts')
print(result)
```
相关问题
python groupby count()
The groupby() function in Python is used to group the data based on a specific column. The count() function is used to count the number of occurrences of each group.
Here is an example of how to use groupby() and count() functions in Python:
```python
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'Name': ['John', 'Mary', 'John', 'Peter', 'Mary'],
'Age': [25, 35, 30, 45, 40],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Miami']})
# group by the 'Name' column and count the number of occurrences
count_df = df.groupby(['Name']).count()
print(count_df)
```
Output:
```
Age City
Name
John 2 2
Mary 2 2
Peter 1 1
```
In the above example, we have grouped the data by the 'Name' column and counted the number of occurrences of each name. The resulting dataframe shows the count for each name.
python groupby agg count
引用\[1\]中的示例代码展示了如何使用groupby和agg函数对DataFrame进行分组和聚合操作。在这个例子中,使用groupby('A')对DataFrame按照列'A'进行分组,然后对不同的列'B'和'C'采用不同的聚合方法,其中'B'列使用了np.mean和np.sum方法,'C'列使用了'count'和np.std方法。最后的结果是每个分组的聚合结果。\[1\]
引用\[2\]中的示例代码展示了如何使用groupby和agg函数对DataFrame进行分组和聚合操作。在这个例子中,使用groupby('A')对DataFrame按照列'A'进行分组,然后对另外一列'B'采用不同的聚合方法,其中使用了np.mean和np.std方法。最后的结果是每个分组的聚合结果。\[2\]
根据你的问题,你想知道如何使用groupby和agg函数计算count。你可以使用groupby函数指定要分组的列,然后使用agg函数指定要对哪一列进行计算。在你的示例中,你可以使用df.groupby('A')\['B'\].agg('count')来计算列'B'的计数。这将返回每个分组中列'B'的计数结果。\[3\]
#### 引用[.reference_title]
- *1* *2* [python中分组函数groupby和分组运算函数agg小结](https://blog.csdn.net/weixin_37536446/article/details/82109431)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [python pandas中groupby()的使用,sum和count](https://blog.csdn.net/qq_39290990/article/details/121435796)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
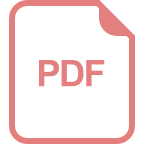
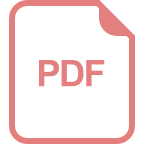
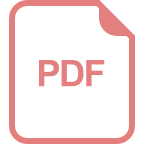













