C语言输入3个学生的信息,放在结构体数组中,要求输出全部学生的信息
时间: 2023-07-14 21:13:56 浏览: 89
可以使用如下的C代码实现:
```c
#include <stdio.h>
struct student {
char name[20];
int age;
float score;
};
int main() {
struct student stu[3];
for (int i = 0; i < 3; i++) {
printf("请依次输入第%d个学生的姓名、年龄和成绩:\n", i+1);
scanf("%s %d %f", stu[i].name, &stu[i].age, &stu[i].score);
}
printf("全部学生的信息如下:\n");
for (int i = 0; i < 3; i++) {
printf("姓名:%s,年龄:%d,成绩:%.2f\n", stu[i].name, stu[i].age, stu[i].score);
}
return 0;
}
```
在上面的代码中,我们定义了一个`struct student`结构体来表示每个学生的信息,包括姓名`name`、年龄`age`和成绩`score`。然后我们定义了一个数组`stu`来存储三个学生的信息,通过循环输入每个学生的信息,并将其存储到数组中。最后,我们再次使用循环输出所有学生的信息。
相关问题
编写程序:有3个学生的信息,放在结构体数组中,要求输出全部学生的信息
### 回答1:
可以使用循环遍历结构体数组,输出每个学生的信息,例如:for (int i = 0; i < 3; i++) { printf("学生%d:%s %d\n", i+1, stu[i].name, stu[i].age); }
### 回答2:
要编写程序输出3个学生的信息,可以使用结构体数组来保存学生的信息。
首先,我们需要定义一个结构体来表示学生的信息。每个学生的信息包括姓名、年龄和学号。可以这样定义结构体:
```c
typedef struct {
char name[20];
int age;
int studentId;
} Student;
```
接下来,我们创建一个包含3个学生的结构体数组,并为每个学生的信息赋值。可以通过循环来完成这个操作:
```c
Student students[3];
for (int i = 0; i < 3; i++) {
printf("请输入第 %d 个学生的姓名:", i+1);
scanf("%s", students[i].name);
printf("请输入第 %d 个学生的年龄:", i+1);
scanf("%d", &students[i].age);
printf("请输入第 %d 个学生的学号:", i+1);
scanf("%d", &students[i].studentId);
}
```
最后,我们使用循环遍历结构体数组,输出每个学生的信息:
```c
for (int i = 0; i < 3; i++) {
printf("第 %d 个学生的姓名:%s\n", i+1, students[i].name);
printf("第 %d 个学生的年龄:%d\n", i+1, students[i].age);
printf("第 %d 个学生的学号:%d\n", i+1, students[i].studentId);
}
```
这样,程序就能输出全部学生的信息了。
### 回答3:
下面是一个用C语言编写的程序,用来存储3个学生的信息,并输出所有学生的信息:
#include <stdio.h>
// 定义学生结构体
struct Student {
char name[20];
int age;
float score;
};
int main() {
// 定义存储3个学生的结构体数组
struct Student students[3];
// 输入学生信息
for (int i = 0; i < 3; i++) {
printf("请输入第%d个学生的姓名:", i+1);
scanf("%s", students[i].name);
printf("请输入第%d个学生的年龄:", i+1);
scanf("%d", &students[i].age);
printf("请输入第%d个学生的分数:", i+1);
scanf("%f", &students[i].score);
}
// 输出学生信息
printf("所有学生的信息如下:\n");
for (int i = 0; i < 3; i++) {
printf("姓名:%s 年龄:%d 分数:%.2f\n", students[i].name, students[i].age, students[i].score);
}
return 0;
}
运行以上程序,会依次提示输入3个学生的姓名、年龄和分数,然后输出所有学生的信息。每个学生的信息包括姓名、年龄和分数。
C语言输入3个学生的编号、姓名、性别、年龄,放在结构体数字组中,要求输出全部学生的信息
以下是一个示例代码,可以实现输入三个学生信息并输出全部学生信息的功能:
```c
#include <stdio.h>
// 定义结构体类型
struct student {
int id;
char name[20];
char gender;
int age;
};
int main() {
// 定义结构体数组
struct student stu[3];
int i;
// 输入学生信息
for (i = 0; i < 3; i++) {
printf("请输入第%d个学生的信息:\n", i + 1);
printf("学号:");
scanf("%d", &stu[i].id);
printf("姓名:");
scanf("%s", stu[i].name);
printf("性别:");
scanf(" %c", &stu[i].gender); // 这里需要注意,要在%c前加一个空格,避免上一个输入的回车被读入
printf("年龄:");
scanf("%d", &stu[i].age);
}
// 输出学生信息
printf("\n所有学生的信息如下:\n");
for (i = 0; i < 3; i++) {
printf("学号:%d\t姓名:%s\t性别:%c\t年龄:%d\n", stu[i].id, stu[i].name, stu[i].gender, stu[i].age);
}
return 0;
}
```
运行程序后,按照提示输入每个学生的信息,最后会输出所有学生的信息。注意,在输入性别时需要在%c前加一个空格,避免上一个输入的回车被读入。
相关推荐
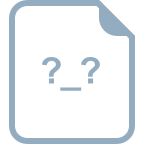
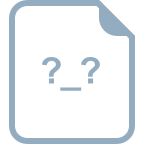







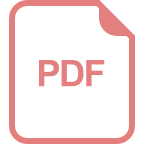
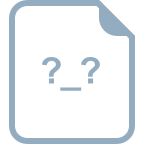
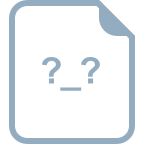