头歌python数字时钟走字
时间: 2023-07-25 18:43:00 浏览: 136
可以使用 Python 中的 time 模块和 tkinter 模块来实现数字时钟走字的效果。以下是一个简单的示例代码:
```python
import time
import tkinter as tk
def update_time():
# 获取当前时间
current_time = time.strftime('%H:%M:%S')
# 更新标签文本
time_label.config(text=current_time)
# 每隔1秒调用一次函数
time_label.after(1000, update_time)
# 创建窗口
window = tk.Tk()
window.title('数字时钟')
window.geometry('200x80')
# 创建标签
time_label = tk.Label(window, text='', font=('Arial', 36))
time_label.pack()
# 开始更新时间
update_time()
# 进入消息循环
window.mainloop()
```
这个程序会创建一个窗口和一个标签,标签会显示当前的时间。每隔1秒钟,程序就会更新一下标签中的文本,从而实现数字时钟走字的效果。你可以根据自己的需要调整字体、窗口大小等参数。
相关问题
python数字时钟走字
好的,让我来为您解答关于Python数字时钟走字的问题。在Python中,我们可以通过time库来控制时间的变化,并结合tkinter库来实现数字时钟的显示。下面是一个简单的示例代码:
```python
import tkinter as tk
import time
def update_clock():
current_time = time.strftime('%H:%M:%S')
clock_label.config(text=current_time)
clock_label.after(1000, update_clock)
root = tk.Tk()
root.title('Digital Clock')
clock_label = tk.Label(root, font=('Arial', 80), bg='black', fg='green')
clock_label.pack(fill='both', expand=True)
update_clock()
root.mainloop()
```
这段代码中,我们定义了一个update_clock()函数,用于每隔1秒更新一次当前时间,并将其显示在tkinter的Label组件中。然后在主循环中调用update_clock()函数,即可实现数字时钟的走字效果。
希望这个回答可以帮助到您。
头歌pythonwhile模拟器猜数字游戏答案
在Python中,你可以使用`while`循环和一些随机数生成来创建一个简单的猜数字游戏。下面是一个基本的模拟器示例:
```python
import random
# 随机生成一个1到100之间的整数作为答案
answer = random.randint(1, 100)
# 初始化猜测次数为0
guesses_taken = 0
print("欢迎来到猜数字游戏!我想了一个1到100之间的整数。")
# 使用while循环直到玩家猜对为止
while True:
# 获取用户输入
guess = int(input("请输入你的猜测:"))
guesses_taken += 1
# 检查猜的数字是否正确
if guess < answer:
print("太小了,再试一次。")
elif guess > answer:
print("太大了,再试试。")
else:
print(f"恭喜,你猜对了!你总共猜了{guesses_taken}次。")
break
```
在这个游戏中,玩家不断输入猜测,程序会根据猜测给出提示,直到猜对答案。
阅读全文
相关推荐
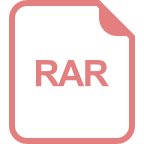
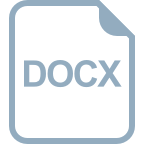













