如何将List<String> 转为Map<Long, String>
时间: 2023-10-14 09:09:19 浏览: 106
可以使用Java 8的Stream API和Collectors工具类来将List<String>转为Map<Long, String>,具体代码如下:
```
List<String> list = Arrays.asList("1:a", "2:b", "3:c");
Map<Long, String> map = list.stream()
.map(s -> s.split(":"))
.collect(Collectors.toMap(
arr -> Long.parseLong(arr[0]),
arr -> arr[1]
));
```
这里先将每个字符串按照":"进行分割,得到一个包含两个元素的数组,然后使用Collectors.toMap方法将这个数组转为Map<Long, String>,其中第一个元素作为键,第二个元素作为值。注意,如果列表中有重复的键,会抛出IllegalStateException异常。如果需要处理重复键的情况,可以使用Collectors.toMap的第三个参数来指定合并函数。
相关问题
List<String>转为List<Long>
将`List<String>`转换为`List<Long>`通常需要遍历字符串列表,然后对每个字符串元素进行解析并将其转换为Long类型。这个过程可以手动完成,也可以使用Java 8及更高版本提供的流(Stream)操作。以下是一个简单的示例:
```java
List<String> stringList = Arrays.asList("1", "2", "3"); // 假设这是你的字符串列表
List<Long> longList = stringList.stream()
.map(Long::valueOf) // 使用Long.valueOf()函数将字符串转换为Long
.collect(Collectors.toList()); // 将流转换回List
```
在这个例子中,`map()`方法会应用到列表中的每个元素,`Long::valueOf`是一个方法引用,它将字符串转换为Long。最后,`collect()`方法收集处理后的结果。
java如何把List<String>转为List<Long>
可以使用Java 8的Stream API来将List<String>转换为List<Long>。以下是示例代码:
```java
List<String> stringList = Arrays.asList("1", "2", "3", "4", "5");
List<Long> longList = stringList.stream()
.map(Long::valueOf)
.collect(Collectors.toList());
```
首先,我们将需要转换的List<String>初始化为一个字符串列表。然后,我们使用Stream API的map()方法将字符串列表中的每个元素转换为Long类型的数字。最后,我们使用collect()方法将结果收集到新的List<Long>中。
请注意,在使用map()方法时,我们使用了Java 8中的方法引用(Long::valueOf),这相当于将每个元素传递给valueOf()方法进行转换。
阅读全文
相关推荐
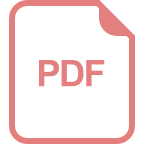
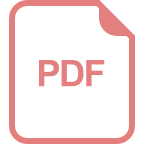














