给定一个字符串 s ,请你找出其中不含有重复字符的 最长子串 的长度。
时间: 2023-02-22 13:00:50 浏览: 130
给定一个字符串 s,要求找出其中不含有重复字符的最长子串的长度。
这是一个经典的字符串问题,可以使用滑动窗口解决。
滑动窗口算法的思路是:
1. 定义两个指针 start 和 end,分别指向子串的开头和结尾。
2. 当 end 指向的字符在子串中出现过,就将 start 指针右移,直到该字符不在子串中。
3. 每次 end 指针右移,都需要更新子串的长度。
4. 重复步骤 2 和 3,直到 end 指针到达字符串末尾。
用代码实现该算法的方法如下:
```
int lengthOfLongestSubstring(string s) {
int start = 0, end = 0, max_len = 0;
unordered_set<char> char_set;
while (end < s.size()) {
if (char_set.count(s[end]) == 0) {
char_set.insert(s[end]);
end++;
max_len = max(max_len, end - start);
} else {
char_set.erase(s[start]);
start++;
}
}
return max_len;
}
```
这是一个时间复杂度为 O(n) 的算法,其中 n 是字符串的长度。
相关问题
给定一个字符串,请你找出其中不含有重复字符的最长子串的长度
题目描述:
给定一个字符串,请你找出其中不含有重复字符的最长子串的长度。
示例 1:
输入: "abcabcbb"
输出: 3
解释: 因为无重复字符的最长子串是 "abc",所以其长度为 3。
示例 2:
输入: "bbbbb"
输出: 1
解释: 因为无重复字符的最长子串是 "b",所以其长度为 1。
示例 3:
输入: "pwwkew"
输出: 3
解释: 因为无重复字符的最长子串是 "wke",所以其长度为 3。
请注意,你的答案必须是 子串 的长度,"pwke" 是一个子序列,不是子串。
解题思路:
使用滑动窗口的思想,维护一个窗口,窗口内的元素不重复,每次移动右边界,如果右边界的元素在窗口内出现过,则移动左边界,直到窗口内不再有重复元素。
代码实现:
给定一个字符串,请你找出其中不含有重复字符的 最长子串 的长度
你的问题可以通过使用滑动窗口算法来解决。具体实现如下:
1. 定义一个字典来存储字符和它们在字符串中的最近一次出现的索引。
2. 定义两个指针 left 和 right,分别指向子串的左右边界。
3. 当右指针指向的字符在字典中已经存在时,更新左指针的位置为该字符在字典中的最近一次出现的索引加1。
4. 在遍历过程中,记录每个不重复子串的最大长度,并返回该长度。
以下是实现代码:
```
def lengthOfLongestSubstring(s: str) -> int:
char_dict = {} # 存储每个字符及其最近一次出现的索引
left = 0 # 左指针,子串左边界
max_len = 0 # 不重复子串的最大长度
for i, char in enumerate(s):
if char in char_dict and char_dict[char] >= left:
left = char_dict[char] + 1
char_dict[char] = i
max_len = max(max_len, i - left + 1)
return max_len
```
你可以使用该函数进行测试:
```
s = "abcabcbb"
print(lengthOfLongestSubstring(s)) # 输出3,对应的不重复子串为"abc"
s = "bbbbb"
print(lengthOfLongestSubstring(s)) # 输出1,对应的不重复子串为"b"
s = "pwwkew"
print(lengthOfLongestSubstring(s)) # 输出3,对应的不重复子串为"wke"
s = ""
print(lengthOfLongestSubstring(s)) # 输出0,空字符串不存在不重复子串
s = " "
print(lengthOfLongestSubstrng(s)) # 输出1,空格""为不重复子串
```
阅读全文
相关推荐
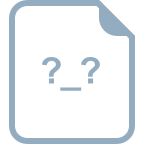









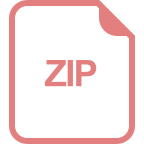
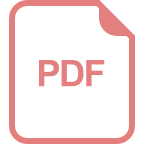