class Complex implements Cloneable{ private double real; private double imaginary; public Complex(){ this.real = 0; this.imaginary = 0; } public Complex(double a){ this.real = a; this.imaginary = 0; } public Complex(double a, double b){ this.real = a; this.imaginary = b; } public double getRealPart(){ return this.real; } public double getImaginaryPart(){ return this.imaginary; } public String toString(){ if(this.imaginary==0){ return this.real + ""; } else if(this.real==0){ return this.imaginary + "i"; } else{ return this.real + " + " + this.imaginary + "i"; } } public Complex add(Complex other){ double newReal = this.real + other.getRealPart(); double newImaginary = this.imaginary + other.getImaginaryPart(); return new Complex(newReal, newImaginary); } public Complex subtract(Complex other){ double newReal = this.real - other.getRealPart(); double newImaginary = this.imaginary - other.getImaginaryPart(); return new Complex(newReal, newImaginary); } public Complex multiply(Complex other){ double newReal = this.real * other.getRealPart() - this.imaginary * other.getImaginaryPart(); double newImaginary = this.real * other.getImaginaryPart() + this.imaginary * other.getRealPart(); return new Complex(newReal, newImaginary); } public Complex divide(Complex other){ double denominator = Math.pow(other.getRealPart(),2) + Math.pow(other.getImaginaryPart(),2); double newReal = (this.real * other.getRealPart() + this.imaginary * other.getImaginaryPart()) / denominator; double newImaginary = (this.imaginary * other.getRealPart() - this.real * other.getImaginaryPart()) / denominator; return new Complex(newReal, newImaginary); } public double abs(){ return Math.sqrt(Math.pow(this.real, 2) + Math.pow(this.imaginary, 2)); } public Object clone; public Object clone() throws CloneNotSupportedException{ return super.clone(); }生成这段代码的uml图
时间: 2024-04-02 20:37:03 浏览: 19
抱歉,由于我是一名语言模型AI,并不能直接生成UML图,但是我可以解释一下这段代码的UML类图:
这段代码实现了一个复数类 Complex,其中包含了实部和虚部两个属性,以及加、减、乘、除、求模等操作的方法。该类还实现了 Cloneable 接口,并重写了 clone() 方法,用于实现对象的深拷贝。
在UML类图中,Complex 类应该是一个具体类(concrete class),并包含了两个属性:real 和 imaginary,分别表示实部和虚部。该类应该还有一个默认的构造函数(Complex())和两个重载构造函数(Complex(double a) 和 Complex(double a, double b)),用于创建 Complex 类的实例对象。
此外,Complex 类还应该实现了一些操作方法,如:
- getRealPart():获取实部值。
- getImaginaryPart():获取虚部值。
- add():实现两个复数的加法操作。
- subtract():实现两个复数的减法操作。
- multiply():实现两个复数的乘法操作。
- divide():实现两个复数的除法操作。
- abs():获取复数的模值。
最后,Complex 类还应该实现了 Cloneable 接口,并重写了 clone() 方法,用于实现对象的深拷贝。
相关问题
分析代码:public class AddStudentViewHandler implements ActionListener { private AddStudentView addStudentView; private StuMainView mainView; public AddStudentViewHandler(AddStudentView addStudentView, StuMainView mainView){ this.addStudentView=addStudentView;
这是一个Java类,名为AddStudentViewHandler,实现了ActionListener接口。
该类有两个私有成员变量,分别是AddStudentView类型的addStudentView和StuMainView类型的mainView。
类中有一个构造函数AddStudentViewHandler,接受两个参数addStudentView和mainView,并将它们分别赋值给成员变量addStudentView和mainView。
该类没有重写ActionListener接口中的任何方法,因此需要根据实际需求来添加对应的方法。
”public class Estimator implements TopItems.Estimator<Long> { private final UserSimilarity userSimilarityImpl; private final long theUserID; private final double minSim; public Estimator(UserSimilarity userSimilarityImpl, long theUserID, double minSim) { this.userSimilarityImpl = userSimilarityImpl; this.theUserID = theUserID; this.minSim = minSim; } @Override public double estimate(Long userID) throws Exception { if (userID == theUserID) { return Double.NaN; } double sim = userSimilarityImpl.userSimilarity(theUserID, userID); return sim >= minSim ? sim : Double.NaN; } }“ 解释代码
这段代码是一个实现了 `TopItems.Estimator` 接口的 Java 类 `Estimator`。该接口用于估算用户之间的相似度,并返回前 n 个最相似的用户。
在类的构造函数中,传入了三个参数:
1. `userSimilarityImpl` 是一个用户相似度的实现类,用于计算两个用户之间的相似度;
2. `theUserID` 是需要计算相似度的用户 ID;
3. `minSim` 是两个用户之间相似度的最小阈值。
在 `estimate` 方法中,传入了一个 `userID` 参数,用于计算 `theUserID` 和 `userID` 之间的相似度。如果 `userID` 等于 `theUserID`,则返回 `Double.NaN`,否则计算出两个用户之间的相似度 `sim`。如果 `sim` 大于等于 `minSim`,则返回 `sim`,否则返回 `Double.NaN`。
相关推荐
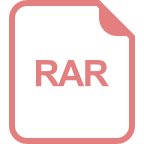
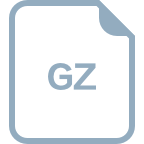
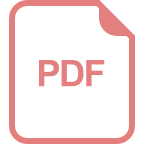













