urlencode编码与解码 C++
时间: 2023-09-07 12:17:11 浏览: 52
在 C 语言中,可以使用以下库函数来进行 urlencode 编码和解码:
1. urlencode 编码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
char* urlencode(char* str) {
int len = strlen(str);
char* encoded = (char*)malloc(len * 3 + 1);
int j = 0;
for (int i = 0; i < len; i++) {
if (isalnum(str[i]) || str[i] == '-' || str[i] == '_' || str[i] == '.' || str[i] == '~') {
encoded[j++] = str[i];
} else if (str[i] == ' ') {
encoded[j++] = '+';
} else {
sprintf(encoded + j, "%%%02X", str[i]);
j += 3;
}
}
encoded[j] = '\0';
return encoded;
}
```
2. urlencode 解码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
char* urldecode(char* str) {
int len = strlen(str);
char* decoded = (char*)malloc(len + 1);
int j = 0;
for (int i = 0; i < len; i++) {
if (str[i] == '+') {
decoded[j++] = ' ';
} else if (str[i] == '%') {
char hex[3] = { str[i + 1], str[i + 2], '\0' };
decoded[j++] = strtol(hex, NULL, 16);
i += 2;
} else {
decoded[j++] = str[i];
}
}
decoded[j] = '\0';
return decoded;
}
```
使用示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char* str = "hello world!";
char* encoded = urlencode(str);
printf("Encoded: %s\n", encoded);
char* decoded = urldecode(encoded);
printf("Decoded: %s\n", decoded);
free(encoded);
free(decoded);
return 0;
}
```
输出结果:
```
Encoded: hello+world%21
Decoded: hello world!
```
相关推荐
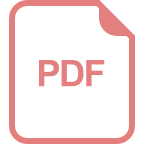














