用C++写一个BF算法
时间: 2023-12-27 07:18:11 浏览: 26
Sure, here's an example Brainfuck algorithm written in C:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_LENGTH 30000 // maximum length of the Brainfuck input string
int main(void) {
char input[MAX_LENGTH]; // input string for Brainfuck
int tape[MAX_LENGTH] = {0}; // initialize the Brainfuck tape with all 0's
int *ptr = tape; // initialize the Brainfuck data pointer to point to the first index of the tape
int loops[MAX_LENGTH] = {0}; // array to store indices of left brackets in the input string
int stack[MAX_LENGTH] = {0}; // stack to keep track of nested loops
int *loop_ptr = loops; // pointer to the current index of the loops array
int stack_top = 0; // index of the top of the stack
int i = 0; // index of the current character of the input string
// prompt the user for a Brainfuck input string
printf("Enter a Brainfuck program:\n");
fgets(input, MAX_LENGTH, stdin);
// process each character of the input string
while (input[i] != '\0') {
switch (input[i]) {
case '>':
ptr++;
break;
case '<':
ptr--;
break;
case '+':
(*ptr)++;
break;
case '-':
(*ptr)--;
break;
case '.':
putchar(*ptr);
fflush(stdout);
break;
case ',':
*ptr = getchar();
break;
case '[':
if (*ptr == 0) {
// skip the loop
i = stack[stack_top] - 1;
stack_top--;
} else {
// add the current index to the loops array and push it onto the stack
*loop_ptr = i;
loop_ptr++;
stack_top++;
stack[stack_top] = i;
}
break;
case ']':
if (*ptr != 0) {
// jump back to the beginning of the loop
i = *(loop_ptr - 1);
} else {
// pop the loop off the stack
loop_ptr--;
stack_top--;
}
break;
}
i++;
}
return 0;
}
```
This program prompts the user for a Brainfuck input string, then executes it using an array of integers as the tape and a pointer to the current data position on the tape. It also handles loops using a stack to keep track of nested brackets. Note that this implementation assumes that the input string contains a valid Brainfuck program and does not perform any error checking.
相关推荐
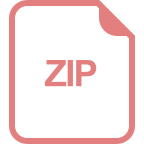














