let arr = [{ name: '青苹果', header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", children: [{ name: "洗发水", header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", children: [{ name: '北方米饭', header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", }, { name: '南方米饭', header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", }] }, { name: "沐浴露", header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", }] }, { name: '红苹果', header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", children: [{ name: "锄头", header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", }, { name: "锤子", header: '"https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200"', comment:"听说今天要使用递归实现评论列表", }] }]使用递归渲染在vue3页面出层级
时间: 2023-11-25 11:36:30 浏览: 37
可以考虑使用Vue3的递归组件来实现树形结构的渲染。
首先,在template中定义一个递归组件,例如:
```
<template>
<div class="comment">
<div class="comment-header">
<img :src="header" alt="header">
<span>{{ name }}</span>
</div>
<div class="comment-content">{{ comment }}</div>
<div class="comment-children">
<comment v-for="child in children" :key="child.name" :data="child"></comment>
</div>
</div>
</template>
<script>
export default {
name: 'comment',
props: {
data: {
type: Object,
required: true
}
}
}
</script>
```
在递归组件中,使用v-for来遍历当前节点的子节点,并将子节点作为递归组件的props传递下去,直到遍历到叶子节点。
然后,在父组件中使用递归组件来渲染整个树形结构,例如:
```
<template>
<div class="comment-list">
<comment v-for="item in arr" :key="item.name" :data="item"></comment>
</div>
</template>
<script>
import Comment from './Comment.vue'
export default {
components: {
Comment
},
data() {
return {
arr: [{
name: '青苹果',
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
children: [{
name: "洗发水",
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
children: [{
name: '北方米饭',
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
}, {
name: '南方米饭',
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
}]
}, {
name: "沐浴露",
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
}]
}, {
name: '红苹果',
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
children: [{
name: "锄头",
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
}, {
name: "锤子",
header: 'https://img0.baidu.com/it/u=1348709467,3751255405&fm=253&fmt=auto&app=138&f=JPEG?w=200&h=200',
comment: '听说今天要使用递归实现评论列表',
}]
}]
}
}
}
</script>
```
在父组件中使用v-for来遍历整个树形结构的根节点,并将根节点作为递归组件的props传递下去。
这样就可以使用递归组件来实现树形结构的渲染了。
相关推荐
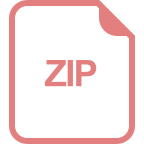














