请用C语言写下面代码,输入一组数查找键值key=8是否在数组中如果存在则输出其索引位置否则输出负一1
时间: 2023-06-01 12:07:48 浏览: 77
这是一段C代码,用来查找键值key=8是否在数组中,如果存在则输出其索引位置,否则输出负一。
```
#include <stdio.h>
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int key = 8;
int n = sizeof(arr) / sizeof(arr[0]);
int i, index = -1;
for (i = 0; i < n; i++) {
if (arr[i] == key) {
index = i;
break;
}
}
if (index != -1) {
printf("Key found at index %d\n", index);
} else {
printf("-1\n");
}
return 0;
}
```
相关问题
c语言用哈希的方法在40、59、84、73、21、17、52、39、74、4、47、65、45、99、19 、97、64、67、85、22、23、83、98、69、88、3、42、60、1、94这三十个数中查找出三数之和等于100的三个数,并输出这三个数和输出其在原数组的索引下标。请给出详细注释
好的,这是一道比较经典的算法题,可以用哈希表来解决。具体步骤如下:
1.先定义一个哈希表,用来存储数组中的数值和对应的下标。
2.遍历数组中的每一个数,在哈希表中查找是否存在另外两个数满足三数之和等于100。
3.如果存在,则输出这三个数和它们在原数组中的下标。
下面是具体的代码实现和注释:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100000 //哈希表最大的大小
typedef struct Node {
int key; //哈希表的键值
int value; //哈希表的值
int index; //该数在原数组中的下标
} Node;
Node hashTable[MAX_SIZE]; //定义一个哈希表
int hashSize = 0; //哈希表当前的大小
//哈希函数,将数字映射到哈希表中
int hash(int key) {
return abs(key) % MAX_SIZE; //取绝对值,防止负数出现
}
//向哈希表中插入一个数
void insert(int key, int value, int index) {
int h = hash(key); //计算哈希值
while (hashTable[h].value != 0) { //如果该位置已经被占用了
h++; //线性探测法,往后找一个空位
if (h == MAX_SIZE) h = 0; //如果到了哈希表的末尾,则从头开始
}
hashTable[h].key = key; //将数值存储在哈希表中
hashTable[h].value = value; //将该数值对应的下标存储在哈希表中
hashTable[h].index = index; //将该数在原数组中的下标存储在哈希表中
hashSize++; //哈希表的大小加1
}
//在哈希表中查找是否存在两个数满足三数之和等于100
int search(int key, int index1, int index2) {
int h = hash(key); //计算哈希值
while (hashTable[h].value != 0) { //如果该位置已经被占用了
if (hashTable[h].key == key && hashTable[h].index != index1 && hashTable[h].index != index2) { //如果找到了符合条件的数
printf("%d + %d + %d = 100\n", hashTable[h].key, hashTable[index1].key, hashTable[index2].key); //输出这三个数
printf("index: %d %d %d\n", hashTable[h].index, hashTable[index1].index, hashTable[index2].index); //输出它们在原数组中的下标
return 1; //返回1,表示找到了
}
h++; //如果没找到,则继续往后找
if (h == MAX_SIZE) h = 0; //如果到了哈希表的末尾,则从头开始
}
return 0; //返回0,表示没找到
}
int main() {
int a[] = {40, 59, 84, 73, 21, 17, 52, 39, 74, 4, 47, 65, 45, 99, 19, 97, 64, 67, 85, 22, 23, 83, 98, 69, 88, 3, 42, 60, 1, 94};
int n = sizeof(a) / sizeof(int); //数组的大小
for (int i = 0; i < n; i++) {
insert(a[i], i + 1, i); //将数组中的每一个数插入到哈希表中
}
for (int i = 0; i < n - 2; i++) { //枚举每一个数
for (int j = i + 1; j < n - 1; j++) { //枚举该数后面的每一个数
int sum = a[i] + a[j]; //计算两个数的和
if (search(100 - sum, i, j)) { //在哈希表中查找是否存在满足条件的数
return 0; //找到了就结束程序
}
}
}
return 0;
}
```
在本题中,由于要输出每个数在原数组中的下标,因此在哈希表中除了要存储数值和值对应的下标,还要额外存储该数在原数组中的下标。在查找满足条件的三个数时,需要注意判断哈希表中找到的数是否和已经枚举出来的两个数的下标相同,避免输出重复的答案。
使用C语言编写程序给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。 你可以假设每种输入只会对应一个答案,并且你不能使用两次相同的元素。 你可以按任意顺序返回答案。输入整数N
在C语言中,可以通过创建一个哈希表来存储遍历数组时的元素值和对应的索引,以实现快速查找和为为目标值的两个整数。下面是一种可能的实现方法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义哈希表节点结构体
typedef struct HashNode {
int key;
int value;
struct HashNode *next;
} HashNode;
// 定义哈希表结构体
typedef struct {
HashNode **buckets;
int size;
} HashTable;
// 创建哈希表
HashTable* createHashTable(int size) {
HashTable *hashTable = (HashTable*)malloc(sizeof(HashTable));
hashTable->size = size;
hashTable->buckets = (HashNode**)malloc(sizeof(HashNode*) * size);
for (int i = 0; i < size; i++) {
hashTable->buckets[i] = NULL;
}
return hashTable;
}
// 释放哈希表内存
void freeHashTable(HashTable *hashTable) {
for (int i = 0; i < hashTable->size; i++) {
HashNode *node = hashTable->buckets[i];
while (node) {
HashNode *temp = node;
node = node->next;
free(temp);
}
}
free(hashTable->buckets);
free(hashTable);
}
// 插入键值对到哈希表
void insert(HashTable *hashTable, int key, int value) {
int index = key % hashTable->size;
HashNode *newNode = (HashNode*)malloc(sizeof(HashNode));
newNode->key = key;
newNode->value = value;
newNode->next = hashTable->buckets[index];
hashTable->buckets[index] = newNode;
}
// 在哈希表中查找键对应的值
int search(HashTable *hashTable, int key) {
int index = key % hashTable->size;
HashNode *node = hashTable->buckets[index];
while (node) {
if (node->key == key) {
return node->value;
}
node = node->next;
}
return -1; // 未找到
}
// 返回数组中两个整数的和为target的下标
int* twoSum(int* nums, int numsSize, int target, int* returnSize) {
HashTable *hashTable = createHashTable(numsSize);
int *result = (int*)malloc(sizeof(int) * 2);
for (int i = 0; i < numsSize; ++i) {
int complement = target - nums[i];
int complementIndex = search(hashTable, complement);
if (complementIndex != -1) {
result[0] = complementIndex;
result[1] = i;
*returnSize = 2;
freeHashTable(hashTable);
return result;
}
insert(hashTable, nums[i], i);
}
*returnSize = 0;
freeHashTable(hashTable);
return result;
}
int main() {
int nums[] = {2, 7, 11, 15};
int target = 9;
int returnSize = 0;
int* indices = twoSum(nums, sizeof(nums) / sizeof(nums[0]), target, &returnSize);
if (returnSize == 2) {
printf("找到的两个数的下标为: [%d, %d]\n", indices[0], indices[1]);
} else {
printf("未找到符合条件的两个数\n");
}
free(indices);
return 0;
}
```
这段代码定义了一个哈希表,并提供了创建、插入和查找的功能。`twoSum`函数利用这个哈希表来快速查找是否存在和为`target`的两个整数。如果找到,返回它们的下标;否则,返回没有找到的信息。
阅读全文
相关推荐
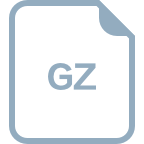















