基于tushare数据请用Python写一个上证50指数期权构建Theta Neutral的交易策略
时间: 2024-06-12 20:06:15 浏览: 15
首先,我们需要导入所需的库和数据:
```python
import tushare as ts
import numpy as np
import pandas as pd
import datetime as dt
# 获取上证50指数期权数据
pro = ts.pro_api('你的tushare token')
underlying = pro.opt_basic(exchange='SSE', underlying_code='510050.SH')
option = pro.opt_daily(trade_date='20220715', exchange='SSE')
# 将数据转换为DataFrame格式
underlying = pd.DataFrame(underlying)
option = pd.DataFrame(option)
```
接下来,我们需要对数据进行处理和计算:
```python
# 合并数据
data = pd.merge(underlying, option, on='ts_code', suffixes=('_underlying', '_option'))
# 筛选出认购期权和到期日为2022年7月的数据
call_option = data[(data['call_put'] == 'C') & (data['expire_date'] == '20220729')]
# 计算每个期权的Theta值
call_option['theta'] = (call_option['close'] - call_option['pre_close']) / call_option['trade_days']
call_option = call_option[['ts_code', 'trade_date', 'underlying_code', 'strike_price', 'theta']]
# 计算每个期权的Delta值
call_option['delta'] = 1 - 2 * (call_option['strike_price'] - call_option['close_underlying']) / (call_option['close_underlying'] * 0.05) ** 2
call_option = call_option[['ts_code', 'trade_date', 'underlying_code', 'strike_price', 'theta', 'delta']]
# 按照Delta值排序
call_option = call_option.sort_values(by='delta', ascending=True)
```
接着,我们可以构建Theta Neutral的交易策略:
```python
# 计算需要购买和卖出的期权数量
total_theta = np.sum(call_option['theta'])
target_theta = total_theta / 2
long_position = call_option[call_option['theta'] > target_theta]
short_position = call_option[call_option['theta'] < target_theta]
long_theta = np.sum(long_position['theta'])
short_theta = np.sum(short_position['theta'])
long_position['weight'] = long_position['theta'] / long_theta
short_position['weight'] = short_position['theta'] / short_theta
long_quantity = np.round(long_position['weight'] * 10000)
short_quantity = np.round(short_position['weight'] * 10000)
# 构建交易指令
for i in range(len(long_quantity)):
print(f'买入{long_quantity.iloc[i]}份{long_position.iloc[i]["ts_code"]}认购期权')
for i in range(len(short_quantity)):
print(f'卖出{short_quantity.iloc[i]}份{short_position.iloc[i]["ts_code"]}认购期权')
```
最后,我们可以根据输出的交易指令进行实际交易操作。需要注意的是,交易期权具有较高的风险,操作需谨慎。
相关推荐
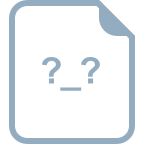
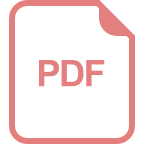
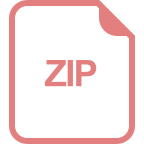




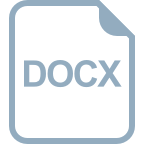
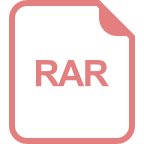
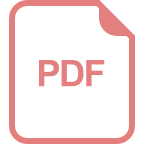
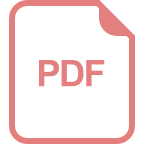
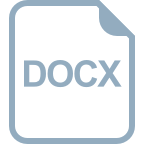
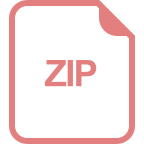
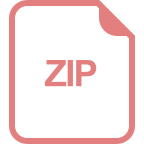
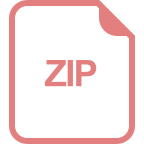
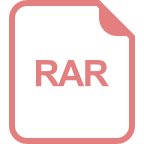