C++中将YUV转换为H265格式
时间: 2023-09-08 13:04:16 浏览: 163
### 回答1:
好的。
C语言中可以使用第三方库来实现YUV格式到H265格式的转换。这些第三方库包括FFmpeg、libx265等。
你可以使用这些库中提供的API来进行格式转换。例如,使用FFmpeg库可以使用函数avcodec_encode_video2()来实现视频编码。
示例代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <libavcodec/avcodec.h>
#include <libavutil/imgutils.h>
int main(int argc, char *argv[])
{
// 初始化FFmpeg库
av_register_all();
// 创建编码器上下文
AVCodec *codec = avcodec_find_encoder(AV_CODEC_ID_H265);
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
// 设置编码参数
codec_ctx->bit_rate = 400000;
codec_ctx->width = 640;
codec_ctx->height = 480;
codec_ctx->time_base = (AVRational){1, 25};
codec_ctx->framerate = (AVRational){25, 1};
codec_ctx->gop_size = 10;
codec_ctx->max_b_frames = 1;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
// 打开编码器
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
fprintf(stderr, "Could not open codec\n");
exit(1);
}
// 分配输出帧
AVFrame *frame = av_frame_alloc();
frame->format = codec_ctx->pix_fmt;
frame->width = codec_ctx->width;
frame->height = codec_ctx->height;
av_frame_get_buffer(frame, 0);
// 填充输入帧数据(此处假设已经从YUV文件中读取到了帧数据)
// ...
// 初始化AVPacket
AVPacket packet;
av_init_packet(&packet);
packet.data = NULL;
### 回答2:
在C语言中将YUV转换为H265格式的过程可以分为以下几个步骤:
1. 导入相关的头文件和库:在C语言中,要进行YUV到H265的转换首先需要导入相关的头文件和库,如#include <stdio.h>和#include <x265.h>。
2. 初始化x265库:调用x265_param_alloc()函数初始化一个x265_param结构体,并设置相关参数。例如,可以设置YUV分辨率、帧率等信息。
3. 打开编码器:使用x265_encoder_open()函数打开编码器,将初始化好的x265_param结构体传递给该函数。
4. 分配输入YUV帧内存:使用malloc()函数动态分配存储YUV帧数据的内存空间。
5. 读取YUV帧数据:从外部读取一帧YUV数据,可以使用文件操作函数,如fread()。
6. 将YUV数据传递给编码器:通过调用x265_encoder_encode()函数,将读取到的YUV数据传递给编码器进行编码。
7. 获取编码后的数据:使用x265_encoder_encode()函数返回的x265_nal结构体指针获取编码后的数据。
8. 将编码后的数据写入文件:使用文件操作函数,如fwrite(),将编码后的数据写入文件。
9. 释放内存和关闭编码器:使用free()函数释放之前分配的内存空间,使用x265_encoder_close()函数关闭编码器。
通过以上步骤,就可以在C语言中将YUV转换为H265格式。当然,在实际操作中还需处理一些错误判断和异常情况,以及对多个YUV帧进行循环编码等。
### 回答3:
在C语言中,将YUV转换为H265格式可以通过使用适当的库和算法来实现。下面是一个简单的示例代码:
1. 首先,包含必要的头文件和库:
```c
#include <stdio.h>
#include <x265.h>
```
2. 定义输入YUV文件和输出H265文件的路径:
```c
#define INPUT_YUV_FILE "input.yuv"
#define OUTPUT_H265_FILE "output.h265"
```
3. 设置编码器参数:
```c
x265_param* param = x265_param_alloc();
x265_param_default(param);
param->sourceWidth = 1920; // 输入YUV的宽度
param->sourceHeight = 1080; // 输入YUV的高度
param->fpsNum = 30; // 帧率的分子
param->fpsDenom = 1; // 帧率的分母
```
4. 打开输入YUV文件和输出H265文件:
```c
FILE* input = fopen(INPUT_YUV_FILE, "rb");
FILE* output = fopen(OUTPUT_H265_FILE, "wb");
```
5. 创建编码器:
```c
x265_encoder* encoder = x265_encoder_open(param);
```
6. 设置编码器的输入参数:
```c
x265_picture* pic_in = x265_picture_alloc();
x265_picture_init(param, pic_in);
pic_in->planes[0] = malloc(param->sourceWidth * param->sourceHeight * sizeof(uint8_t)); // Y分量
pic_in->planes[1] = malloc(param->sourceWidth * param->sourceHeight / 4 * sizeof(uint8_t)); // U分量
pic_in->planes[2] = malloc(param->sourceWidth * param->sourceHeight / 4 * sizeof(uint8_t)); // V分量
```
7. 循环读取输入YUV文件的帧,并编码为H265格式:
```c
while (/* 读取输入YUV文件的条件 */) {
/* 读取一帧YUV数据到pic_in中 */
x265_nal* nals;
uint32_t num_nals;
x265_encoder_encode(encoder, &nals, &num_nals, pic_in, NULL);
/* 将编码后的nals写入输出H265文件中 */
}
```
8. 关闭文件和释放内存:
```c
fclose(input);
fclose(output);
free(pic_in->planes[0]);
free(pic_in->planes[1]);
free(pic_in->planes[2]);
x265_picture_free(pic_in);
x265_encoder_close(encoder);
x265_param_free(param);
```
上述代码只是一个简单示例,实际操作中可能还需要添加错误处理和其他参数配置等。在实际使用中,可以参考x265库提供的API文档和示例代码来进行更详细的操作和配置。
阅读全文
相关推荐
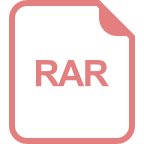
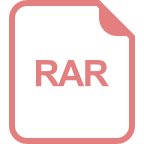
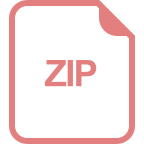
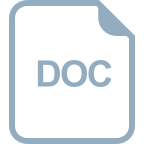
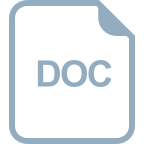
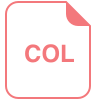





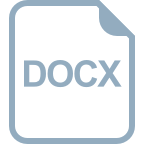
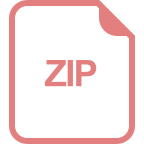
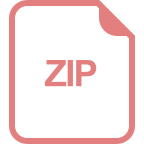
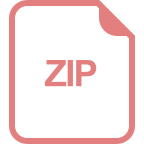