java建立一棵二叉排序树
时间: 2023-07-06 09:34:38 浏览: 83
好的,以下是Java实现二叉排序树的代码:
```java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
public TreeNode(int val) {
this.val = val;
this.left = null;
this.right = null;
}
}
class BST {
private TreeNode root;
public BST() {
root = null;
}
public void insert(int val) {
root = insertNode(root, val);
}
private TreeNode insertNode(TreeNode root, int val) {
if (root == null) {
root = new TreeNode(val);
return root;
}
if (val < root.val) {
root.left = insertNode(root.left, val);
} else if (val > root.val) {
root.right = insertNode(root.right, val);
}
return root;
}
public void inorderTraversal() {
inorder(root);
}
private void inorder(TreeNode root) {
if (root != null) {
inorder(root.left);
System.out.print(root.val + " ");
inorder(root.right);
}
}
}
public class Main {
public static void main(String[] args) {
BST bst = new BST();
bst.insert(50);
bst.insert(30);
bst.insert(20);
bst.insert(40);
bst.insert(70);
bst.insert(60);
bst.insert(80);
bst.inorderTraversal();
}
}
```
这个代码实现了二叉排序树的插入和中序遍历,你可以根据需要进行修改和扩展。
相关推荐


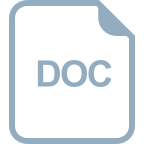
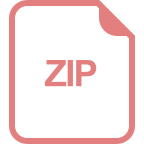

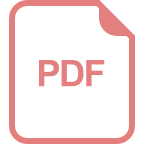

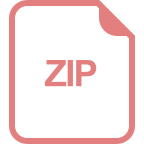
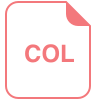

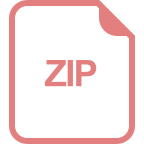
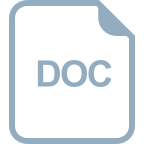
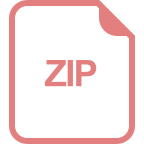
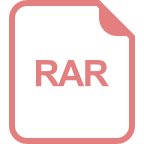
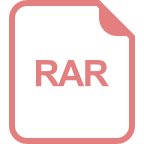
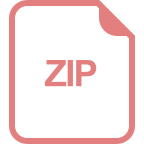