x_image = tf.reshape(xs, [-1, 28, 28, 1]) 的-1,代表什么含义?可以改为NONE?
时间: 2023-10-05 17:12:10 浏览: 56
-1在reshape函数中代表一个占位符,其值由计算机自动计算得出,使得reshape函数能够根据其他参数的维度推断出-1所代表的维度大小。因此,可以将-1理解为一个“不确定”的维度。在本例中,-1是为了让reshape函数能够根据原始数据包含的元素数量自动计算出剩余的维度大小。因为原始数据包含28 * 28个元素,所以可以用-1代替剩余的维度。在这个特定的例子中,可以将-1改为None或其他正整数也可以实现相同的效果。
相关问题
xs = tf.placeholder(tf.float32, [None, 784], name='x_input') x_image = tf.reshape(xs, [-1, 28, 28, 1]) 中的reshape起到了什么作用?
reshape的作用是将输入的数据xs按照给定的形状[-1, 28, 28, 1]进行重新排列,并返回新的tensor x_image。在这个例子中,reshape函数的参数中[-1, 28, 28, 1]表示将xs分成若干个28×28大小的图片,每个图片有1个通道。这个重新排列后的tensor可以作为神经网络的输入。
基于tensorflow使用lenet-5实现对mnist手写数字识别分类
好的,以下是基于TensorFlow使用LeNet-5实现对MNIST手写数字识别分类的步骤:
1. 导入必要的库
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
```
2. 加载MNIST数据集
```python
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
```
3. 定义网络结构
```python
# 定义输入和输出的placeholder
x = tf.placeholder(tf.float32, [None, 784])
y_ = tf.placeholder(tf.float32, [None, 10])
# 将输入向量转换为28x28的图像
x_image = tf.reshape(x, [-1, 28, 28, 1])
# 定义第一个卷积层
conv1_weights = tf.Variable(tf.truncated_normal([5, 5, 1, 6], stddev=0.1))
conv1_biases = tf.Variable(tf.constant(0.1, shape=[6]))
conv1 = tf.nn.conv2d(x_image, conv1_weights, strides=[1, 1, 1, 1], padding='SAME')
conv1 = tf.nn.relu(conv1 + conv1_biases)
# 定义第一个池化层
pool1 = tf.nn.max_pool(conv1, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME')
# 定义第二个卷积层
conv2_weights = tf.Variable(tf.truncated_normal([5, 5, 6, 16], stddev=0.1))
conv2_biases = tf.Variable(tf.constant(0.1, shape=[16]))
conv2 = tf.nn.conv2d(pool1, conv2_weights, strides=[1, 1, 1, 1], padding='VALID')
conv2 = tf.nn.relu(conv2 + conv2_biases)
# 定义第二个池化层
pool2 = tf.nn.max_pool(conv2, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME')
# 将第二个池化层的输出展开为一维向量
pool2_flat = tf.reshape(pool2, [-1, 5 * 5 * 16])
# 定义第一个全连接层
fc1_weights = tf.Variable(tf.truncated_normal([5 * 5 * 16, 120], stddev=0.1))
fc1_biases = tf.Variable(tf.constant(0.1, shape=[120]))
fc1 = tf.nn.relu(tf.matmul(pool2_flat, fc1_weights) + fc1_biases)
# 定义第二个全连接层
fc2_weights = tf.Variable(tf.truncated_normal([120, 84], stddev=0.1))
fc2_biases = tf.Variable(tf.constant(0.1, shape=[84]))
fc2 = tf.nn.relu(tf.matmul(fc1, fc2_weights) + fc2_biases)
# 定义输出层
fc3_weights = tf.Variable(tf.truncated_normal([84, 10], stddev=0.1))
fc3_biases = tf.Variable(tf.constant(0.1, shape=[10]))
y = tf.matmul(fc2, fc3_weights) + fc3_biases
```
4. 定义损失函数和优化器
```python
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y_, logits=y))
train_step = tf.train.AdamOptimizer(1e-4).minimize(cross_entropy)
```
5. 定义评估模型的准确率
```python
correct_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
```
6. 启动会话并训练模型
```python
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(20000):
batch_xs, batch_ys = mnist.train.next_batch(64)
sess.run(train_step, feed_dict={x: batch_xs, y_: batch_ys})
if i % 1000 == 0:
train_accuracy = accuracy.eval(feed_dict={x: batch_xs, y_: batch_ys})
print('step %d, training accuracy %g' % (i, train_accuracy))
test_accuracy = accuracy.eval(feed_dict={x: mnist.test.images, y_: mnist.test.labels})
print('test accuracy %g' % test_accuracy)
```
以上就是基于TensorFlow使用LeNet-5实现对MNIST手写数字识别分类的全部步骤。
相关推荐
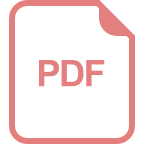
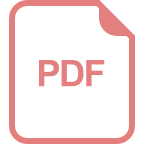
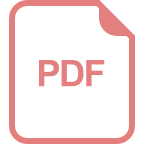









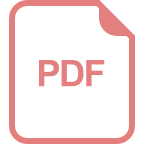
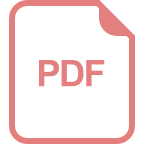
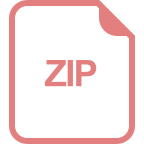
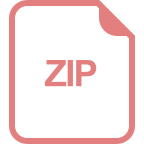