python OpenCV 皮带边缘检测
时间: 2023-10-31 16:07:21 浏览: 43
以下是使用Python OpenCV进行皮带边缘检测的一些基本步骤:
1. 导入Python OpenCV库和NumPy库:
```python
import cv2
import numpy as np
```
2. 读取图像:
```python
img = cv2.imread('belt.jpg', 0)
```
3. 应用高斯滤波器平滑图像:
```python
blur = cv2.GaussianBlur(img, (5,5), 0)
```
4. 应用Canny边缘检测算法:
```python
edges = cv2.Canny(blur, 50, 150, apertureSize=3)
```
5. 对图像进行形态学操作:
```python
kernel = np.ones((5,5), np.uint8)
dilation = cv2.dilate(edges, kernel, iterations=1)
```
6. 寻找轮廓并绘制:
```python
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, contours, -1, (0,255,0), 3)
```
完整代码示例:
```python
import cv2
import numpy as np
img = cv2.imread('belt.jpg', 0)
blur = cv2.GaussianBlur(img, (5,5), 0)
edges = cv2.Canny(blur, 50, 150, apertureSize=3)
kernel = np.ones((5,5), np.uint8)
dilation = cv2.dilate(edges, kernel, iterations=1)
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, contours, -1, (0,255,0), 3)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:在运行以上代码时,请确保已经安装了Python OpenCV库和NumPy库。
相关推荐
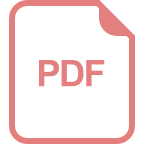
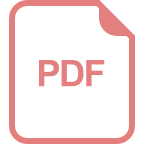














