tcp校验和c语言代码
时间: 2023-05-15 08:06:08 浏览: 136
以下是一个计算TCP校验和的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <netinet/in.h>
unsigned short checksum(unsigned short *buf, int nwords)
{
unsigned long sum;
for(sum=0; nwords>0; nwords--)
sum += *buf++;
sum = (sum >> 16) + (sum &0xffff);
sum += (sum >> 16);
return (unsigned short)(~sum);
}
int main()
{
char data[] = "Hello, world!";
int datalen = strlen(data);
char src_ip[] = "192.168.0.1";
char dst_ip[] = "192.168.0.2";
unsigned short src_port = 1234;
unsigned short dst_port = 5678;
char packet[4096];
memset(packet, 0, sizeof(packet));
struct iphdr *iph = (struct iphdr *)packet;
struct tcphdr *tcph = (struct tcphdr *)(packet + sizeof(struct iphdr));
iph->ihl = 5;
iph->version = 4;
iph->tos = 0;
iph->tot_len = sizeof(struct iphdr) + sizeof(struct tcphdr) + datalen;
iph->id = htons(54321);
iph->frag_off = 0;
iph->ttl = 255;
iph->protocol = IPPROTO_TCP;
iph->check = 0;
iph->saddr = inet_addr(src_ip);
iph->daddr = inet_addr(dst_ip);
tcph->source = htons(src_port);
tcph->dest = htons(dst_port);
tcph->seq = htonl(1);
tcph->ack_seq = 0;
tcph->doff = 5;
tcph->fin = 0;
tcph->syn = 1;
tcph->rst = 0;
tcph->psh = 0;
tcph->ack = 0;
tcph->urg = 0;
tcph->window = htons(5840);
tcph->check = 0;
tcph->urg_ptr = 0;
memcpy(packet + sizeof(struct iphdr) + sizeof(struct tcphdr), data, datalen);
tcph->check = checksum((unsigned short *)(packet + sizeof(struct iphdr)), sizeof(struct tcphdr) + datalen);
printf("TCP checksum: 0x%x\n", tcph->check);
return 0;
}
```
这个代码可以计算TCP报文的校验和,其中包括IP头和TCP头,以及TCP数据。你可以将需要发送的数据放在`data`数组中,将源IP地址和目的IP地址放在`src_ip`和`dst_ip`字符串中,将源端口和目的端口放在`src_port`和`dst_port`变量中。最终计算出的校验和会被存放在`tcph->check`变量中。
相关推荐
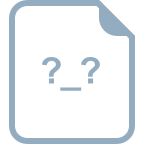
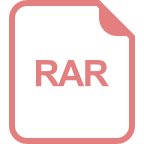














