使用JFrame创建登录窗口(可参考下图或自行设计布局) 当点击登录按钮时,对输入的用户名为和密码进行验证: 当输入的用户为admin,密码为1234时,弹出消息对话框,提示信息为:“用户名和密码正确”,否则,提示信息为:“用户名或密码错误”。 点击重置按钮时: 用户名文本框和密码文本框的内容被清空。
时间: 2024-03-16 13:47:48 浏览: 146
好的,我已经明白了你的问题。下面是一个简单的实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class LoginFrame extends JFrame implements ActionListener {
private JTextField usernameField;
private JPasswordField passwordField;
public LoginFrame() {
setTitle("登录窗口");
setSize(300, 200);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridLayout(3, 2, 5, 5));
panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
JLabel usernameLabel = new JLabel("用户名:");
usernameField = new JTextField();
JLabel passwordLabel = new JLabel("密 码:");
passwordField = new JPasswordField();
JButton loginButton = new JButton("登录");
loginButton.addActionListener(this);
JButton resetButton = new JButton("重置");
resetButton.addActionListener(this);
panel.add(usernameLabel);
panel.add(usernameField);
panel.add(passwordLabel);
panel.add(passwordField);
panel.add(loginButton);
panel.add(resetButton);
add(panel);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("登录")) {
String username = usernameField.getText();
String password = new String(passwordField.getPassword());
if (username.equals("admin") && password.equals("1234")) {
JOptionPane.showMessageDialog(this, "用户名和密码正确");
} else {
JOptionPane.showMessageDialog(this, "用户名或密码错误");
}
} else if (e.getActionCommand().equals("重置")) {
usernameField.setText("");
passwordField.setText("");
}
}
public static void main(String[] args) {
new LoginFrame();
}
}
```
这个实现中,我们使用了`JFrame`来创建窗口,`JPanel`来布局,`JLabel`、`JTextField`、`JPasswordField`、`JButton`等组件来构建界面。当点击登录按钮时,会读取用户名和密码文本框的内容,进行比对并弹出相应的消息对话框;当点击重置按钮时,会清空用户名和密码文本框的内容。
阅读全文
相关推荐
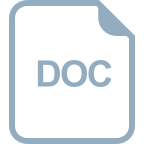
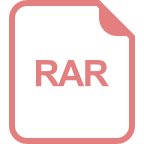
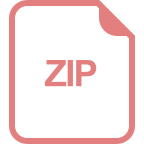


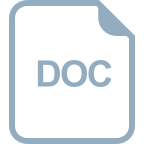
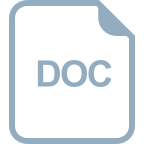
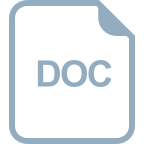
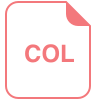
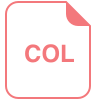
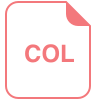







