Java编程题目描述】:某公司举行职员足球比赛,编写程序用于记录球员的信息(队名、球员姓名、球员号码)和比赛进球数。实现随着比赛的推进,每进一球循环输入进球的队名、球员号码。最后输出该项足球比赛的球员进球情况和金靴奖得主。
时间: 2024-03-18 10:40:53 浏览: 229
好的,这是一道比较典型的Java编程题目。我可以给您提供一份代码参考,您可以根据实际情况进行修改和完善。
```java
import java.util.*;
class Player {
String teamName;
String playerName;
int playerNumber;
int goals;
public Player(String teamName, String playerName, int playerNumber) {
this.teamName = teamName;
this.playerName = playerName;
this.playerNumber = playerNumber;
this.goals = 0;
}
public void addGoal() {
this.goals++;
}
public String toString() {
return "Team: " + teamName + ", Player Name: " + playerName + ", Player Number: " + playerNumber + ", Goals: " + goals;
}
}
public class FootballMatch {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
HashMap<Integer, Player> playerMap = new HashMap<>();
while (true) {
System.out.print("Enter team name: ");
String teamName = sc.nextLine();
System.out.print("Enter player name: ");
String playerName = sc.nextLine();
System.out.print("Enter player number: ");
int playerNumber = Integer.parseInt(sc.nextLine());
Player player = new Player(teamName, playerName, playerNumber);
playerMap.put(playerNumber, player);
System.out.print("Add more players? (y/n): ");
String input = sc.nextLine();
if (input.equals("n")) {
break;
}
}
System.out.println("Ready to start the match!");
while (true) {
System.out.print("Enter team name that scored a goal: ");
String teamName = sc.nextLine();
System.out.print("Enter player number that scored a goal: ");
int playerNumber = Integer.parseInt(sc.nextLine());
Player player = playerMap.get(playerNumber);
if (player != null && player.teamName.equals(teamName)) {
player.addGoal();
System.out.println("Goal recorded!");
} else {
System.out.println("Invalid team name or player number!");
}
System.out.print("Add more goals? (y/n): ");
String input = sc.nextLine();
if (input.equals("n")) {
break;
}
}
System.out.println("Match ended!");
int maxGoals = 0;
ArrayList<Player> winners = new ArrayList<>();
for (Map.Entry<Integer, Player> entry : playerMap.entrySet()) {
Player player = entry.getValue();
System.out.println(player);
if (player.goals > maxGoals) {
maxGoals = player.goals;
winners.clear();
winners.add(player);
} else if (player.goals == maxGoals) {
winners.add(player);
}
}
System.out.print("Golden Boot winners: ");
for (Player player : winners) {
System.out.print(player.playerName + " ");
}
}
}
```
在上面的代码中,我们定义了一个Player类来保存球员信息,包括队名、球员姓名、球员号码和进球数。我们使用HashMap来存储每个球员对象,以便能够方便地通过球员号码来查找球员。
在程序运行时,我们首先让用户输入球员信息,然后循环输入每个进球的队名和球员号码,直到比赛结束。最后,我们将输出每个球员的进球情况以及金靴奖得主。
希望这份代码可以帮助到您,如有不清楚的地方,可以随时向我提问。
阅读全文
相关推荐





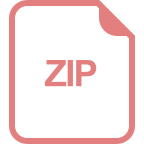







