生成一段代码,用python编写,爬取网页上每条新闻的标题,作者,时间
时间: 2023-09-25 13:06:26 浏览: 85
### 回答1:
import requests
from bs4 import BeautifulSoup# 获取网页源码
resp = requests.get('http://xxxxx.com/news')# 使用BeautifulSoup解析网页
soup = BeautifulSoup(resp.text, 'lxml')# 遍历每一条新闻
for item in soup.find_all('div', class_='news-item'):
# 获取标题
title = item.find('h3').string
# 获取作者
author = item.find('span', class_='news-item-author').string
# 获取时间
time = item.find('span', class_='news-item-time').string
print(title, author, time)
### 回答2:
import requests
from bs4 import BeautifulSoup
def scrape_news(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
news_titles = soup.find_all('a', class_='title') # 获取新闻标题的<a>标签
news_authors = soup.find_all('div', class_='author') # 获取新闻作者的<div>标签
news_times = soup.find_all('div', class_='time') # 获取新闻发布时间的<div>标签
for title, author, time in zip(news_titles, news_authors, news_times):
news_title = title.get_text() # 提取新闻标题文本
news_author = author.get_text() # 提取新闻作者文本
news_time = time.get_text() # 提取新闻发布时间文本
print(f"标题: {news_title}\n作者: {news_author}\n时间: {news_time}\n")
url = "https://www.example.com/news" # 你要爬取的网页链接
scrape_news(url)
### 回答3:
以下是一个用Python编写的代码,可以爬取网页上每条新闻的标题、作者和时间:
```python
import requests
from bs4 import BeautifulSoup
# 定义要爬取的网页链接
url = "http://example.com/news" # 将example.com/news替换为实际的新闻网页链接
# 发送请求,获取网页内容
response = requests.get(url)
content = response.text
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(content, "html.parser")
# 找到所有新闻文章的父标签
news_articles = soup.find_all("div", {"class": "news-article"})
# 遍历每个新闻文章,提取标题、作者和时间
for article in news_articles:
# 提取标题
title = article.find("h2").text
# 提取作者
author = article.find("span", {"class": "author"}).text
# 提取时间
time = article.find("span", {"class": "time"}).text
# 打印结果
print("标题:", title)
print("作者:", author)
print("时间:", time)
print("-------------")
```
请注意,上述代码中的`url`变量需要替换为实际的新闻网页链接,同时需要安装`requests`和`beautifulsoup4`库。此代码使用`requests`库发送网络请求,`BeautifulSoup`库解析网页内容,通过标签和类名来定位新闻文章的标题、作者和时间。最后,通过循环将每个新闻文章的相关信息打印出来。
阅读全文
相关推荐
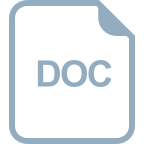
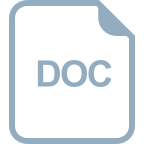
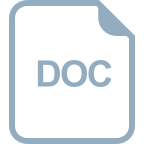

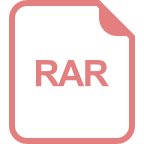
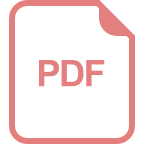
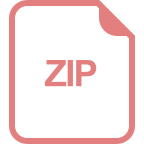
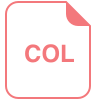
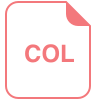
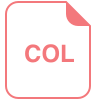
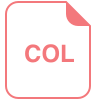
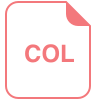
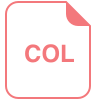
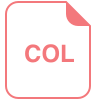
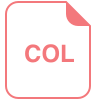
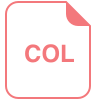
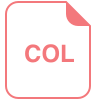
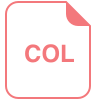
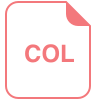