用c++实现大地坐标与空间直角坐标的转化
时间: 2023-11-13 18:06:11 浏览: 190
大地坐标(经纬度)与空间直角坐标(地心坐标)之间的转换涉及到大地椭球模型和大地坐标系的定义。以下是一个简单的C++示例代码,用于实现大地坐标与空间直角坐标的转换:
```cpp
#include <iostream>
#include <cmath>
const double PI = 3.141592653589793238463;
// 定义大地椭球模型参数
const double a = 6378137.0; // 长半轴
const double b = 6356752.3142; // 短半轴
const double f = (a - b) / a; // 扁率
// 定义空间直角坐标结构体
struct CartesianCoordinate {
double x;
double y;
double z;
};
// 经纬度转弧度
double deg2rad(double degree) {
return degree * PI / 180.0;
}
// 大地坐标转空间直角坐标
CartesianCoordinate geodeticToCartesian(double latitude, double longitude, double height) {
double latRad = deg2rad(latitude);
double lonRad = deg2rad(longitude);
double sinLat = std::sin(latRad);
double cosLat = std::cos(latRad);
double sinLon = std::sin(lonRad);
double cosLon = std::cos(lonRad);
double N = a / std::sqrt(1 - f * f * sinLat * sinLat);
CartesianCoordinate result;
result.x = (N + height) * cosLat * cosLon;
result.y = (N + height) * cosLat * sinLon;
result.z = (N * (1 - f * f) + height) * sinLat;
return result;
}
// 空间直角坐标转大地坐标
void cartesianToGeodetic(const CartesianCoordinate& cartesian, double& latitude, double& longitude, double& height) {
double p = std::sqrt(cartesian.x * cartesian.x + cartesian.y * cartesian.y);
double theta = std::atan2(cartesian.z * a, p * b);
latitude = std::atan2(cartesian.z + f * f * b * std::pow(std::sin(theta), 3),
p - a * std::pow(std::cos(theta), 3));
longitude = std::atan2(cartesian.y, cartesian.x);
height = p / std::cos(latitude) - a / std::sqrt(1 - f * f * std::pow(std::sin(latitude), 2));
}
int main() {
// 大地坐标转空间直角坐标
double latitude = 39.9087; // 纬度(单位:度)
double longitude = 116.3975; // 经度(单位:度)
double height = 50.0; // 高程(单位:米)
CartesianCoordinate cartesian = geodeticToCartesian(latitude, longitude, height);
std::cout << "空间直角坐标:\n";
std::cout << "X: " << cartesian.x << "\n";
std::cout << "Y: " << cartesian.y << "\n";
std::cout << "Z: " << cartesian.z << "\n";
// 空间直角坐标转大地坐标
double lat, lon, h;
cartesianToGeodetic(cartesian, lat, lon, h);
std::cout << "大地坐标:\n";
std::cout << "纬度: " << lat << " 度\n";
std::cout << "经度: " << lon << " 度\n";
std::cout << "高程: " << h << " 米\n";
return 0;
}
```
这段代码实现了大地坐标(经纬度)与空间直角坐标(地心坐标)之间的转换。你可以将待转换的经纬度和高程输入到`geodeticToCartesian`函数中,即可得到对应的空间直角坐标。同样地,你也可以输入空间直角坐标到`cartesianToGeodetic`函数中,即可得到对应的大地坐标。
阅读全文
相关推荐
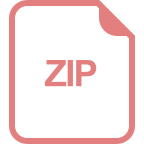
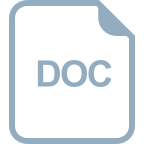

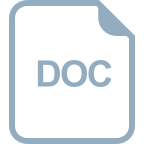

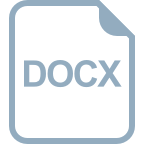




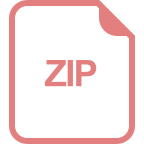
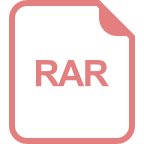



