用Python写一个牛顿插值程序
时间: 2023-04-08 11:03:29 浏览: 107
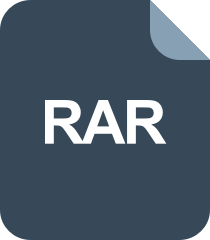
牛顿插值编程

可以的,以下是一个简单的Python牛顿插值程序:
```python
def newton_interpolation(x, y, x0):
n = len(x)
if n != len(y):
raise ValueError("x and y must have the same length")
# 初始化差商表
f = [[0] * n for i in range(n)]
for i in range(n):
f[i][0] = y[i]
# 构造差商表
for j in range(1, n):
for i in range(n - j):
f[i][j] = (f[i + 1][j - 1] - f[i][j - 1]) / (x[i + j] - x[i])
# 计算插值多项式
p = f[0][0]
for j in range(1, n):
t = 1
for i in range(j):
t *= (x0 - x[i])
p += f[0][j] * t
return p
```
其中,x和y分别是已知的数据点的横纵坐标,x0是要求插值的点的横坐标。函数返回插值多项式在x0处的函数值。
阅读全文
相关推荐
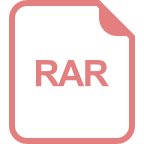
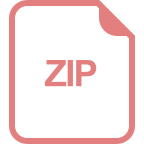


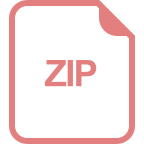
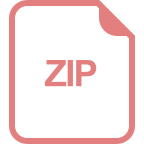
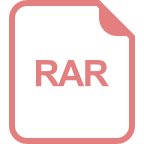







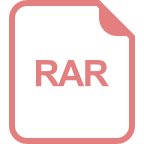
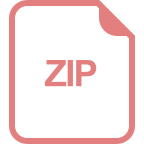