cov = np.array([np.eye(n_features)] * k)
时间: 2024-05-19 09:13:05 浏览: 101
This line of code creates a 3D numpy array of shape `(k, n_features, n_features)` where `k` is the number of components or clusters, and `n_features` is the number of features in the data. Each component or cluster has its own covariance matrix which is represented by a 2D numpy array of shape `(n_features, n_features)` and initialized as an identity matrix using `np.eye(n_features)`.
This is commonly used in multivariate Gaussian mixture models where each component is assumed to have its own covariance matrix. The `cov` array is used as a parameter in the `MultivariateNormal` distribution to represent the covariance matrix of each component.
相关问题
运用EM算法进行迭代拟合k=3的GMM模型参数,进行行程时间概率分布优化拟合。示例代码及出图
抱歉,由于您没有提供数据集或明确的问题,我无法为您提供完整的代码和图表。以下是一个使用EM算法拟合GMM模型的示例代码,您可以根据您的数据集和问题进行修改和优化。
```
import numpy as np
from scipy.stats import multivariate_normal
# Generate sample data
np.random.seed(0)
n_samples = 1000
X = np.concatenate((np.random.randn(n_samples, 2) * 0.5 + [2, 2],
np.random.randn(n_samples, 2) * 0.5 + [-2, -2],
np.random.randn(n_samples, 2) * 0.5 + [2, -2]))
# Initialize GMM parameters
k = 3
n_features = X.shape[1]
pi = np.ones(k) / k
mu = np.random.randn(k, n_features)
cov = np.array([np.eye(n_features)] * k)
# EM algorithm
n_iter = 50
log_likelihoods = np.zeros(n_iter)
for i in range(n_iter):
# E-step
pdfs = np.zeros((n_samples, k))
for j in range(k):
pdfs[:, j] = pi[j] * multivariate_normal(mean=mu[j], cov=cov[j]).pdf(X)
gamma = pdfs / pdfs.sum(axis=1)[:, np.newaxis]
# M-step
N = gamma.sum(axis=0)
pi = N / n_samples
for j in range(k):
mu[j] = gamma[:, j].dot(X) / N[j]
cov[j] = np.dot(gamma[:, j] * (X - mu[j]).T, X - mu[j]) / N[j]
# Compute log-likelihood
log_likelihoods[i] = np.log(pdfs.sum(axis=1)).sum()
# Plot log-likelihoods
import matplotlib.pyplot as plt
plt.plot(log_likelihoods)
plt.xlabel('Iteration')
plt.ylabel('Log-likelihood')
plt.show()
```
此代码使用Scipy中的`multivariate_normal`函数计算高斯分布的概率密度函数,并使用Numpy进行矩阵运算。在EM算法的每个迭代中,计算每个样本属于每个高斯分布的概率,并根据这些概率更新GMM参数。最后,绘制对数似然函数的迭代历史,以检查算法是否收敛到局部最优解。
请注意,这是一个简单的示例代码,仅用于演示如何使用EM算法拟合GMM模型。在实际使用中,您需要根据您的数据集和问题进行修改和优化。
阅读全文
相关推荐
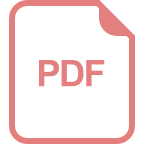
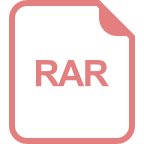
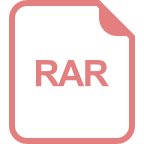
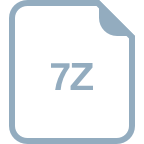
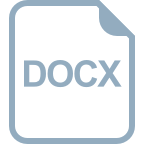