某学校有3类员工:教师、行政人员、教师兼行政人员,共有的信息包括编号、姓名、性别和职工类别。工资计算方法如下。 教师:基本工资+课时数×课时补贴。 行政人员:基本工资+行政补贴。 教师兼行政人员:基本工资+课时数×课时补贴+行政补贴。 分析以上信息,定义人员抽象类,派生不同类型的员工,并完成工资的计算。
时间: 2023-05-27 19:06:50 浏览: 50
人员抽象类的定义:
```
abstract class Employee {
protected int id; // 编号
protected String name; // 姓名
protected char gender; // 性别
public Employee(int id, String name, char gender) {
this.id = id;
this.name = name;
this.gender = gender;
}
public abstract double getSalary(); // 获取工资的抽象方法
public String toString() {
return "编号:" + id + ",姓名:" + name + ",性别:" + gender;
}
}
```
教师类的定义:
```
class Teacher extends Employee {
private int courseHours; // 课时数
private double courseAllowance; // 课时补贴
public Teacher(int id, String name, char gender, int courseHours, double courseAllowance) {
super(id, name, gender);
this.courseHours = courseHours;
this.courseAllowance = courseAllowance;
}
public double getSalary() {
return courseHours * courseAllowance;
}
public String toString() {
return super.toString() + ",职工类别:教师";
}
}
```
行政人员类的定义:
```
class Administrator extends Employee {
private double adminAllowance; // 行政补贴
public Administrator(int id, String name, char gender, double adminAllowance) {
super(id, name, gender);
this.adminAllowance = adminAllowance;
}
public double getSalary() {
return adminAllowance;
}
public String toString() {
return super.toString() + ",职工类别:行政人员";
}
}
```
教师兼行政人员类的定义:
```
class TeacherAdministrator extends Employee {
private int courseHours; // 课时数
private double courseAllowance; // 课时补贴
private double adminAllowance; // 行政补贴
public TeacherAdministrator(int id, String name, char gender, int courseHours, double courseAllowance, double adminAllowance) {
super(id, name, gender);
this.courseHours = courseHours;
this.courseAllowance = courseAllowance;
this.adminAllowance = adminAllowance;
}
public double getSalary() {
return courseHours * courseAllowance + adminAllowance;
}
public String toString() {
return super.toString() + ",职工类别:教师兼行政人员";
}
}
```
测试代码:
```
public static void main(String[] args) {
Employee[] employees = new Employee[3];
employees[0] = new Teacher(1001, "张三", '男', 20, 50);
employees[1] = new Administrator(1002, "李四", '女', 1000);
employees[2] = new TeacherAdministrator(1003, "王五", '男', 30, 60, 1200);
for (Employee employee : employees) {
System.out.println(employee.toString() + ",工资:" + employee.getSalary());
}
}
```
输出结果:
```
编号:1001,姓名:张三,性别:男,职工类别:教师,工资:1000.0
编号:1002,姓名:李四,性别:女,职工类别:行政人员,工资:1000.0
编号:1003,姓名:王五,性别:男,职工类别:教师兼行政人员,工资:3000.0
```
相关推荐
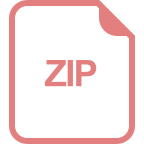
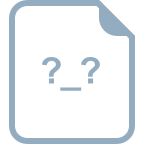
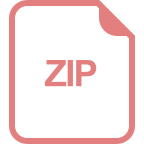














