Linux 平台下,如何让字符设备驱动支持阻塞与非阻塞 IO 模型,以 read 操 作为例,编写代码简要说明
时间: 2024-01-10 12:02:29 浏览: 52
要让字符设备驱动支持阻塞与非阻塞 IO 模型,需要在驱动的 file_operations 结构体中实现对应的函数,如 read 函数。下面是一个简单的示例代码,演示如何在 Linux 平台下实现阻塞与非阻塞 IO 模型。
```
#include <linux/module.h>
#include <linux/fs.h>
#include <linux/uaccess.h>
#include <linux/wait.h>
#define BUF_SIZE 1024
static char buffer[BUF_SIZE];
static int buffer_size = 0;
static DECLARE_WAIT_QUEUE_HEAD(read_wait_queue);
static ssize_t chardev_read(struct file *filp, char __user *buf, size_t count, loff_t *f_pos)
{
int ret;
if (buffer_size == 0) {
if (filp->f_flags & O_NONBLOCK) {
return -EAGAIN;
} else {
// 阻塞等待数据
wait_event_interruptible(read_wait_queue, buffer_size > 0);
}
}
ret = copy_to_user(buf, buffer, buffer_size);
if (ret) {
return -EFAULT;
}
// 读取完毕,清空缓冲区
buffer_size = 0;
return count;
}
static ssize_t chardev_write(struct file *filp, const char __user *buf, size_t count, loff_t *f_pos)
{
int ret;
if (count > BUF_SIZE) {
return -EINVAL;
}
ret = copy_from_user(buffer, buf, count);
if (ret) {
return -EFAULT;
}
buffer_size = count;
// 唤醒阻塞等待数据的进程
wake_up_interruptible(&read_wait_queue);
return count;
}
static struct file_operations chardev_fops = {
.owner = THIS_MODULE,
.read = chardev_read,
.write = chardev_write,
};
static int __init chardev_init(void)
{
int ret;
ret = register_chrdev(0, "chardev", &chardev_fops);
if (ret) {
printk(KERN_ERR "Failed to register chardev\n");
return ret;
}
printk(KERN_INFO "Chardev module loaded\n");
return 0;
}
static void __exit chardev_exit(void)
{
unregister_chrdev(0, "chardev");
printk(KERN_INFO "Chardev module unloaded\n");
}
module_init(chardev_init);
module_exit(chardev_exit);
```
在这个示例代码中,我们实现了一个简单的字符设备驱动,支持阻塞与非阻塞 IO 模型。read 函数中,如果缓冲区中没有数据,而且打开文件时设置了 O_NONBLOCK 标志,那么直接返回 -EAGAIN,表示没有数据可读。如果没有设置 O_NONBLOCK 标志,则调用 wait_event_interruptible 函数阻塞等待数据到来,并通过 wake_up_interruptible 函数唤醒阻塞等待数据的进程。
需要注意的是,这只是一个简单的示例代码,实际上要实现一个完整的字符设备驱动还需要考虑很多其他的问题,比如并发访问、设备文件的创建与销毁等等。
相关推荐
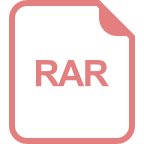
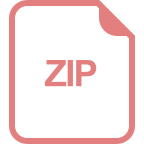
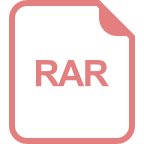














