C++判断map中是否包含某个键
时间: 2024-03-03 22:52:09 浏览: 113
在C++中,可以使用STL库中的`map`来存储键值对数据,判断`map`中是否包含某个键的方式有以下两种:
1. 使用`find()`函数查找键
```c++
#include <map>
#include <iostream>
int main() {
// 定义一个map
std::map<int, std::string> my_map;
// 插入数据
my_map[1] = "apple";
my_map[2] = "banana";
my_map[3] = "orange";
// 判断是否包含某个键
if (my_map.find(2) != my_map.end()) {
std::cout << "my_map contains key 2" << std::endl;
} else {
std::cout << "my_map does not contain key 2" << std::endl;
}
return 0;
}
```
2. 使用`count()`函数统计键的个数
```c++
#include <map>
#include <iostream>
int main() {
// 定义一个map
std::map<int, std::string> my_map;
// 插入数据
my_map[1] = "apple";
my_map[2] = "banana";
my_map[3] = "orange";
// 判断是否包含某个键
if (my_map.count(2) > 0) {
std::cout << "my_map contains key 2" << std::endl;
} else {
std::cout << "my_map does not contain key 2" << std::endl;
}
return 0;
}
```
这两种方式都可以判断`map`中是否包含某个键,但是使用`find()`函数返回一个迭代器,可以直接判断是否等于`end()`,而使用`count()`函数返回键的个数,需要判断是否大于0。
阅读全文
相关推荐
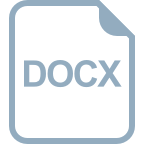







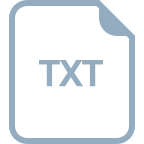
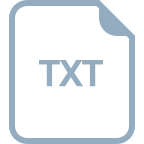
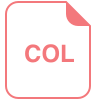
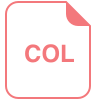
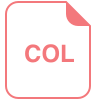




