告诉我C++map的所有用法,写出完整代码
时间: 2023-08-12 21:15:10 浏览: 95
好的,以下是C++中map的常用用法和完整示例代码:
1. 插入元素
`map`中插入元素有多种方式,常用的有以下两种:
```cpp
// 通过下标访问方式插入元素
map<int, string> myMap;
myMap[1] = "one";
myMap[2] = "two";
myMap[3] = "three";
// 通过insert函数插入元素
map<int, string> myMap;
myMap.insert(pair<int, string>(1, "one"));
myMap.insert(make_pair(2, "two"));
myMap.insert(map<int, string>::value_type(3, "three"));
```
2. 遍历元素
```cpp
// 遍历map的所有元素(使用迭代器)
for (auto it = myMap.begin(); it != myMap.end(); it++) {
cout << "key: " << it->first << ", value: " << it->second << endl;
}
// 遍历map的所有元素(使用auto关键字)
for (auto& it : myMap) {
cout << "key: " << it.first << ", value: " << it.second << endl;
}
```
3. 查找元素
```cpp
// 通过下标访问方式查找元素
cout << myMap[1] << endl;
// 通过find函数查找元素
auto it = myMap.find(1);
if (it != myMap.end()) {
cout << "key: " << it->first << ", value: " << it->second << endl;
} else {
cout << "not found" << endl;
}
```
4. 删除元素
```cpp
// 通过下标访问方式删除元素
myMap.erase(1);
// 通过迭代器删除元素
auto it = myMap.find(2);
if (it != myMap.end()) {
myMap.erase(it);
}
// 删除map中所有元素
myMap.clear();
```
5. 其他常用操作
```cpp
// 判断map是否为空
bool empty = myMap.empty();
// 获取map中元素的个数
int size = myMap.size();
// 判断map中是否包含某个元素
bool contains = myMap.count(1) > 0;
```
完整示例代码:
```cpp
#include <iostream>
#include <map>
#include <string>
using namespace std;
int main() {
// 定义一个map,以int类型作为key,string类型作为value
map<int, string> myMap;
// 插入元素
myMap[1] = "one";
myMap[2] = "two";
myMap[3] = "three";
myMap.insert(pair<int, string>(4, "four"));
myMap.insert(make_pair(5, "five"));
// 遍历元素
for (auto& it : myMap) {
cout << "key: " << it.first << ", value: " << it.second << endl;
}
// 查找元素
cout << myMap[1] << endl;
auto it = myMap.find(2);
if (it != myMap.end()) {
cout << "key: " << it->first << ", value: " << it->second << endl;
} else {
cout << "not found" << endl;
}
// 删除元素
myMap.erase(1);
it = myMap.find(2);
if (it != myMap.end()) {
myMap.erase(it);
}
myMap.clear();
// 其他常用操作
bool empty = myMap.empty();
int size = myMap.size();
bool contains = myMap.count(1) > 0;
return 0;
}
```
阅读全文
相关推荐
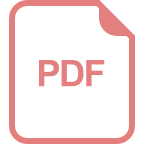




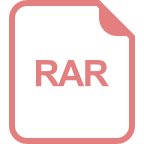
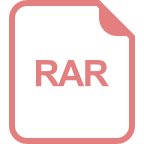
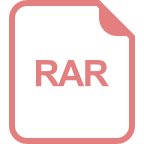
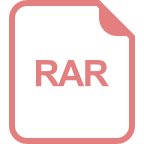
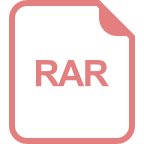
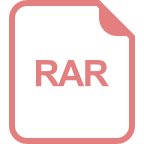
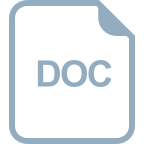
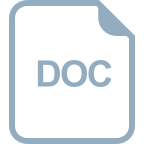
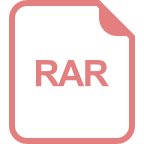
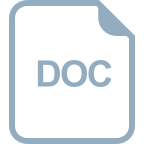
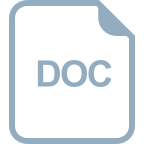
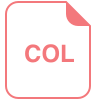